[设计型]编程实现:输入某年某月某日,判断这一天是这一年的第 几天。c语言编程
时间: 2024-10-22 16:22:33 浏览: 15
在C语言中,我们可以使用基姆拉尔森计算公式(Zeller's congruence)来计算闰年的日期。这个算法用于确定给定日期是否为当年的第几天。对于一般的非闰年,我们可以通过从1月1日开始逐日加法来计算。下面是基于这两种情况的一个简单设计:
```cpp
#include <iostream>
// 判断是否为闰年
bool is_leap_year(int year) {
if (year % 4 != 0) return false;
if (year % 100 != 0) return true;
if (year % 400 != 0) return false;
return true;
}
// 计算指定日期是一年中的第几天
int day_of_year(int year, int month, int day) {
// 检查输入合法性
if (month < 1 || month > 12 || day < 1 || day > 31) {
std::cerr << "Invalid date!" << std::endl;
return -1; // 返回错误标记
}
// 根据月份和是否闰年来计算
int days_in_month[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if (is_leap_year(year)) days_in_month[1] = 29;
int total_days = 0;
for (int i = 0; i < month - 1; i++) {
total_days += days_in_month[i];
}
total_days += day;
// 调整闰年的2月
if (month == 2 && is_leap_year(year)) {
total_days--;
}
return total_days;
}
int main() {
int year, month, day;
std::cout << "Enter a year, month (1-12), and day: ";
std::cin >> year >> month >> day;
int result = day_of_year(year, month, day);
if (result != -1) {
std::cout << "The date is the " << result << "th day of the year." << std::endl;
}
return 0;
}
```
在这个程序中,`is_leap_year`函数检查输入的年份是否为闰年,`day_of_year`函数则根据月份和是否闰年来累加前面的天数,最后返回总天数。
阅读全文
相关推荐
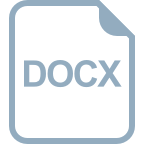
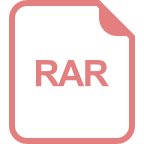
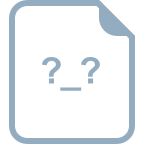



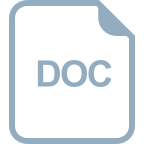
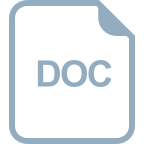
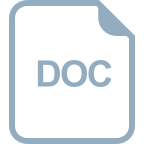
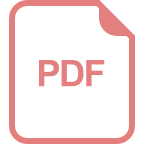
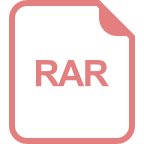
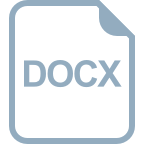
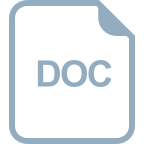
