huffman树的构建C++
时间: 2024-12-04 08:27:49 浏览: 12
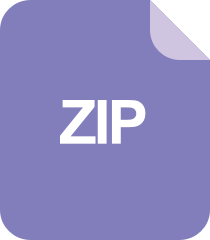
HuffmanTree.zip_数据结构_Visual_C++_
Huffman树,也称为最优二叉树或霍夫曼编码树,是一种特殊的二叉树,用于数据压缩。它的构建通常通过一种贪心算法实现,步骤如下:
1. **计算频率**:首先对每个字符统计其出现的频率。
2. **创建初始集合**:将所有字符及其频率作为节点放入一个优先队列(如最小堆),根节点的权值设为0。
3. **合并节点**:从队列中取出两个权值最小的节点,合并成一个新的节点,新节点的权值等于两子节点权值之和,并将新节点放回队列。
4. **重复直到只剩下一个节点**:这个剩余的节点就是Huffman树的根,表示的是频率最高的字符,叶子节点则是原始字符。
5. **构造编码表**:从根到每个叶子节点,记录下经过的边,形成一个编码表,叶子节点的编码就是从根到该节点的路径上"1"和"0"的组合。
在C++中,你可以使用`std::priority_queue`(最小堆)来实现这一过程。这里是一个简单的伪代码示例:
```cpp
#include <queue>
#include <map>
struct Node {
char data;
int freq;
Node* left, *right;
double weight; // 权值
};
// 添加频率和数据到堆
void insert(Node*& root, char data, int freq) {
if (!root)
root = new Node{data, freq, nullptr, nullptr};
else {
std::pair<Node*, bool> inserted(root, false);
while (inserted.first->freq + inserted.second * freq > root->freq) {
inserted = {inserted.first->left == nullptr ? inserted.first : inserted.first->right, !inserted.second};
}
if (inserted.second)
inserted.first->right = new Node{data, freq, nullptr, nullptr};
else
inserted.first->left = new Node{data, freq, nullptr, nullptr};
}
}
// 构建Huffman树
Node* buildHuffmanTree(std::map<char, int>& freqs) {
std::priority_queue<std::pair<Node*, int>, std::vector<std::pair<Node*, int>>, std::greater<std::pair<Node*, int>>> pq(freqs.begin(), freqs.end());
return build(pq);
}
// ... 其他辅助函数,如build()、encode等
阅读全文
相关推荐
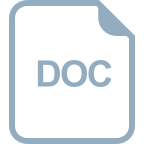
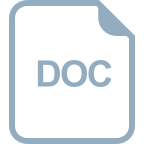
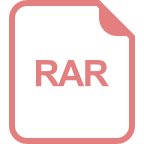

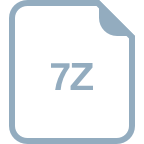
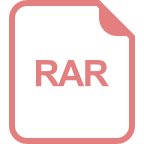
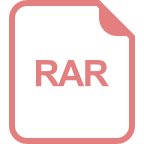
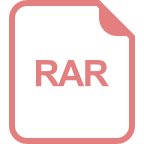
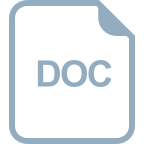
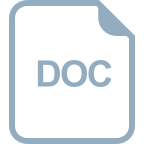








