c++在三角形类 tri 实现两个函数, 功能是输入三个顶点坐标判断是否构成等边三角形
时间: 2024-09-26 13:15:08 浏览: 63
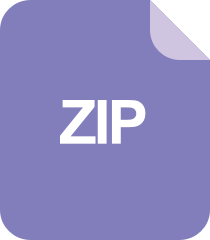
Calculo-triangulo-area:用简单的方法计算三角形的面积,只用整数
在 C++ 中,你可以创建一个名为 "Tri" 的三角形类,其中包含三个顶点属性,并实现 `isEquilateral()` 和 `areVerticesValid()` 函数来判断给定的三个顶点是否构成等边三角形。首先,确保三角形类有一个结构体表示顶点,然后提供必要的构造函数、获取和设置顶点的方法。以下是简单的实现:
```cpp
#include <iostream>
#include <cmath>
// 定义顶点结构
struct Point {
double x, y;
};
class Tri {
private:
Point vertices[3]; // 三个顶点
public:
// 构造函数,接收三个顶点坐标
Tri(double v1x, double v1y, double v2x, double v2y, double v3x, double v3y) {
vertices[0] = {v1x, v1y};
vertices[1] = {v2x, v2y};
vertices[2] = {v3x, v3y};
}
// 获取顶点
Point& getVertex(int index) {
return vertices[index];
}
// 判断是否为等边三角形
bool isEquilateral() const {
for (int i = 0; i < 3; ++i) {
if (std::fabs(vertices[(i + 1) % 3].x - vertices[i].x) !=
std::fabs(vertices[(i + 2) % 3].x - vertices[i].x) ||
std::fabs(vertices[(i + 1) % 3].y - vertices[i].y) !=
std::fabs(vertices[(i + 2) % 3].y - vertices[i].y)) {
return false;
}
}
return true;
}
// 检查顶点是否有效(避免重复或共线)
bool areVerticesValid() const {
if (vertices[0].x == vertices[1].x && vertices[1].x == vertices[2].x || // 同一直线
vertices[0].y == vertices[1].y && vertices[1].y == vertices[2].y) {
return false;
}
double crossProduct = (vertices[1].y - vertices[0].y) * (vertices[2].x - vertices[0].x) -
(vertices[1].x - vertices[0].x) * (vertices[2].y - vertices[0].y);
return crossProduct != 0; // 非零交叉积表明三点不共线
}
};
int main() {
Tri triangle(0, 0, 3, 0, 0, 3); // 示例三角形,三个顶点在同一水平线上
if (triangle.isEquilateral() && triangle.areVerticesValid()) {
std::cout << "This is an equilateral triangle." << std::endl;
} else {
std::cout << "Not an equilateral triangle or vertices are invalid." << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
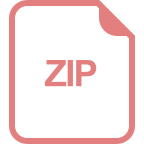
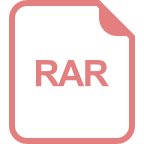




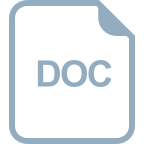










