通讯录项目c语言代码
时间: 2024-09-16 09:02:23 浏览: 49
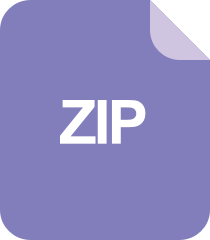
c语言通讯录项目.zip

在C语言中实现一个简单的通讯录项目,我们可以创建一个结构体来存储联系人信息,然后使用数组或链表来管理这些数据。以下是一个简化的示例,展示了如何创建一个静态数组存储联系人:
```c
#include <stdio.h>
#include <string.h>
// 定义联系人结构体
struct Contact {
char name[50];
char phone[20];
};
// 定义联系人数组,假设最大容量为3
#define MAX_CONTACTS 3
struct Contact contacts[MAX_CONTACTS];
// 函数来添加新的联系人
void addContact(char* name, char* phone) {
if (sizeof(contacts) / sizeof(contacts[0]) < MAX_CONTACTS) {
strcpy(contacts[sizeof(contacts) - 1].name, name);
strcpy(contacts[sizeof(contacts) - 1].phone, phone);
printf("Contact added.\n");
} else {
printf("Contacts list is full.\n");
}
}
// 显示所有联系人
void displayContacts() {
for (int i = 0; i < MAX_CONTACTS; i++) {
printf("%s: %s\n", contacts[i].name, contacts[i].phone);
}
}
int main() {
// 添加一些联系人
addContact("Alice", "1234567890");
addContact("Bob", "9876543210");
// 显示所有的联系人
displayContacts();
return 0;
}
```
阅读全文
相关推荐
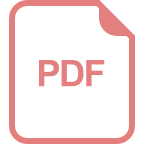
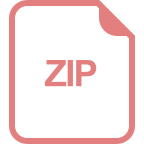

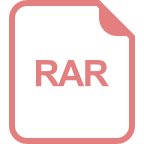
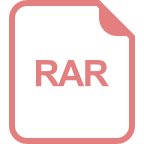
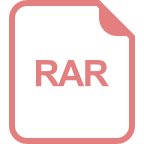
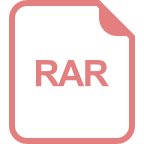
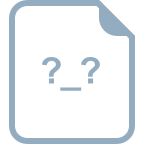
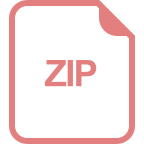
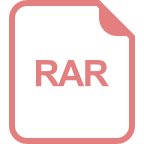
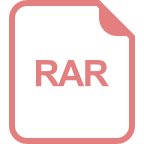
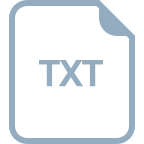
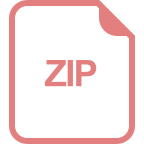
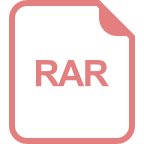
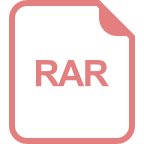
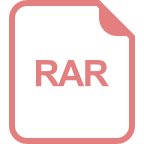
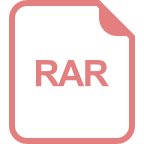