一个类来描述屏幕上一个点,该类的构成包括点的x和y两个坐标,以及一些对点进行的操作,包括:取得点的坐标值,对点的坐标进行赋值。编写应用程序生成该类的对象并对其进行操作,用Java实现
时间: 2024-10-22 10:15:22 浏览: 26
在Java中,我们可以创建一个名为`Point`的简单类来描述屏幕上的一个点。这个类可能会包含两个私有成员变量`x`和`y`,分别表示点的横纵坐标,并提供对应的getter和setter方法以便获取和设置坐标。此外,我们还可以添加一些基本的方法,如复制构造函数、equals()和toString()等。以下是`Point`类的一个基础实现:
```java
public class Point {
private double x;
private double y;
// 构造函数,初始化坐标
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// Getter方法,获取x坐标
public double getX() {
return x;
}
// Setter方法,设置x坐标
public void setX(double newX) {
x = newX;
}
// 获取y坐标
public double getY() {
return y;
}
// 设置y坐标
public void setY(double newY) {
y = newY;
}
// 复制构造函数,用于创建新点的深拷贝
public Point(Point other) {
this.x = other.getX();
this.y = other.getY();
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Point point = (Point) obj;
return Double.compare(point.x, x) == 0 && Double.compare(point.y, y) == 0;
}
@Override
public String toString() {
return "Point{" +
"x=" + x +
", y=" + y +
'}';
}
}
// 示例:创建点对象并进行操作
public static void main(String[] args) {
Point p1 = new Point(1.0, 2.0);
System.out.println("p1: " + p1); // 输出:p1: Point{x=1.0, y=2.0}
p1.setX(3.0);
p1.setY(4.0);
System.out.println("After setting: " + p1); // 输出:After setting: Point{x=3.0, y=4.0}
Point p2 = new Point(p1); // 使用复制构造函数创建新点
System.out.println("p2 is equal to p1? " + p2.equals(p1)); // 输出:p2 is equal to p1? true
}
```
阅读全文
相关推荐
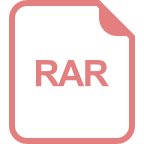
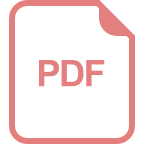
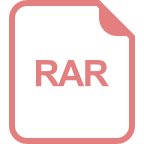













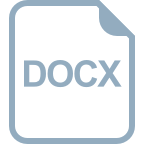