编写程序,检查字符串“Life is short, I use python.”中是否包含“python”,如果包含则替换为“PYTHON”后输出新字符串,否则输出原字符串。
时间: 2024-09-06 14:06:20 浏览: 138
在Python中,你可以使用内置的`replace()`函数轻松地完成这个任务。下面是一个简单的示例程序:
```python
def check_and_replace(input_string, target, replacement):
if target in input_string:
new_string = input_string.replace(target, replacement)
return new_string
else:
return input_string
input_str = "Life is short, I use python."
target_word = "python"
replacement_word = "PYTHON"
output_string = check_and_replace(input_str, target_word, replacement_word)
print(output_string)
```
在这个程序中,`check_and_replace`函数会查找输入字符串`input_string`中是否包含目标字符串`target_word`,如果包含,它会用`replacement_word`替换所有的目标字符串,并返回新的字符串;如果不包含,就直接返回原始字符串。
运行此程序后,如果"python"存在,将会输出:"Life is short, I use PYTHON.",否则就是原来的句子。
相关问题
编写程序,检查字符串"Life is short.I use python"中是否包含字符串"python",若包含则替换为"Python"
是的,字符串"Life is short.I use python"包含字符串"python",可以使用Python代码将它替换为"Python"。以下是示例代码:
```python
string = "Life is short.I use python"
if "python" in string:
string = string.replace("python", "Python")
print(string)
```
输出结果是:"Life is short.I use Python"。
编写程序,检查字符串" Life is short. I use python"中是否包含字符串"python",若包含则替换为"Python"后输出新字符串,否则输出原字符串
在Python中,你可以使用`str.replace()`方法或者切片操作来实现这个功能。下面是两种方式的代码示例:
```python
# 方法一:使用replace()函数
input_string = "Life is short. I use python"
if "python" in input_string:
output_string = input_string.replace("python", "Python")
else:
output_string = input_string
print(output_string)
# 方法二:使用切片操作
output_string = input_string if "python" not in input_string else input_string[:input_string.index("python")] + "Python" + input_string[input_string.index("python") + len("python"):]
print(output_string)
阅读全文
相关推荐
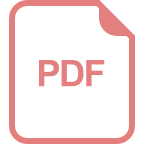
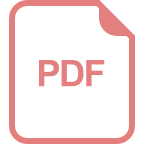
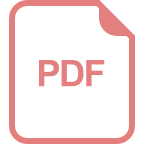














