java复制文件到文件夹
时间: 2024-08-14 21:03:54 浏览: 41
在Java中,复制文件到文件夹可以通过`java.io.File`和`java.nio.file.Files`类提供的方法来实现。这里是一个简单的示例:
```java
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileCopyExample {
public static void main(String[] args) {
// 原始文件路径
String sourceFilePath = "path/to/source/file.txt";
// 目标文件夹路径
String targetDirectoryPath = "path/to/destination/folder";
try {
// 获取源文件对象
File sourceFile = new File(sourceFilePath);
// 创建目标目录(如果不存在)
if (!new File(targetDirectoryPath).exists() && !new File(targetDirectoryPath).mkdirs()) {
System.out.println("Failed to create the target directory.");
return;
}
// 构建完整的目标文件路径
String targetFilePath = Paths.get(targetDirectoryPath, sourceFile.getName()).toString();
// 复制文件
Path destination = Paths.get(targetFilePath);
Files.copy(sourceFile.toPath(), destination, StandardCopyOption.REPLACE_EXISTING); // 如果目标文件已存在,会覆盖
System.out.println("File copied successfully from " + sourceFilePath + " to " + targetFilePath);
} catch (Exception e) {
System.err.println("An error occurred while copying the file: " + e.getMessage());
e.printStackTrace();
}
}
}
```
在这个代码片段中,我们首先创建`File`对象表示源文件,然后检查并创建目标文件夹(如果不存在)。接着,我们将源文件复制到目标文件夹中的相同名称的新文件,如果目标文件已经存在,我们会用`StandardCopyOption.REPLACE_EXISTING`选项替换掉。
阅读全文
相关推荐
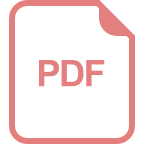
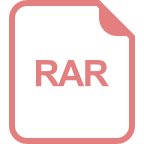
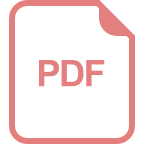
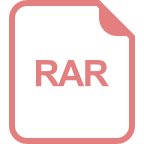
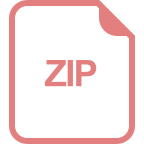
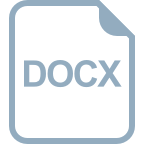
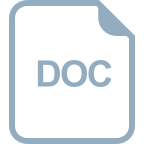
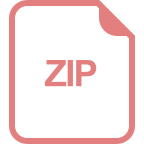
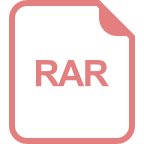
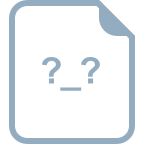
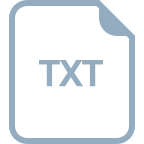
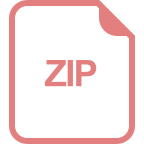
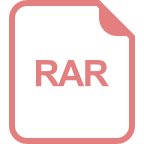
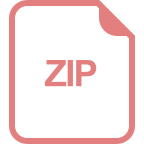
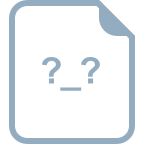
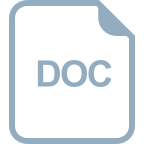