opencv生成椭圆mat
时间: 2024-12-06 11:33:10 浏览: 11
OpenCV是一个开源的计算机视觉库,用于图像处理和计算机视觉任务。如果你想使用OpenCV生成一个椭圆矩阵(Mat),可以按照以下步骤操作:
1. **导入所需模块**:
首先,你需要导入`cv2`模块,它是OpenCV的主要接口。
```python
import cv2
import numpy as np
```
2. **创建椭圆参数**:
定义椭圆中心 `(cx, cy)`、半长轴 `a` 和半短轴 `b`,以及旋转角度 `angle`(如果需要倾斜)。例如:
```python
center = (100, 100) # 椭圆中心
axes = (50, 30) # 半长轴和半短轴
angle = 0 # 旋转角度,0表示水平方向
# 计算旋转矩阵
rot_mat = cv2.getRotationMatrix2D(center, angle, 1)
```
3. **绘制椭圆到矩阵**:
使用 `getRectSubPix` 函数从原点填充椭圆到一个新的 Mat 对象中,因为`drawContours`直接在原始图像上绘图。
```python
ellipse_size = 200 # 椭圆尺寸
ellipse_image = np.zeros((ellipse_size, ellipse_size), dtype=np.uint8)
# 生成椭圆
ellipse_img = cv2.getRectSubPix(ellipse_image, axes, center)
```
4. **应用旋转**:
应用旋转矩阵到椭圆图像。
```python
ellipse_img_rotated = cv2.warpAffine(ellipse_img, rot_mat, (ellipse_size, ellipse_size))
```
5. **结果展示**:
最后,你可以将这个椭圆图像叠加到其他图像上,或者单独显示。
```python
# 将椭圆添加到原始图像上,假设你已经有了一个叫做 "image" 的 Mat
result = cv2.addWeighted(image, 1, ellipse_img_rotated, 0.5, 0)
# 显示结果
cv2.imshow("Ellipse", result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
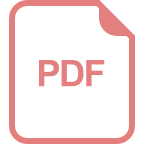
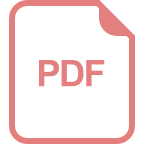
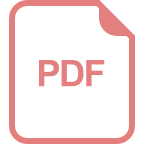
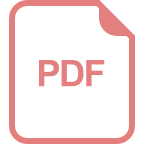
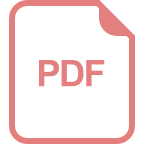
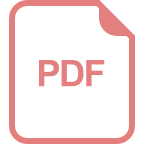
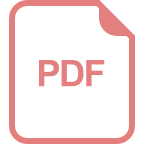
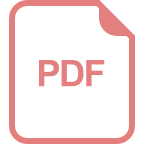
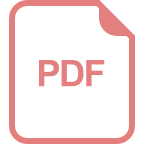
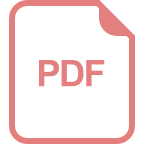
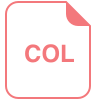
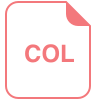
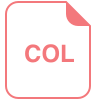
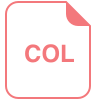



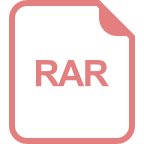
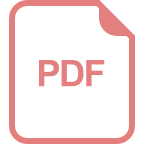