springboot+elementui开发cms内容管理系统的代码
时间: 2023-12-11 07:05:41 浏览: 39
对于使用Spring Boot和Element UI开发CMS内容管理系统的代码,以下是一个简单的示例供你参考:
首先,创建一个Spring Boot项目并添加相应的依赖。在pom.xml文件中添加以下依赖:
```xml
<dependencies>
<!-- Spring Boot Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- Element UI -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>element-ui</artifactId>
<version>2.15.1</version>
</dependency>
<!-- MySQL Connector -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
```
然后,创建一个实体类来表示CMS内容:
```java
@Entity
@Table(name = "cms_content")
public class CmsContent {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
private String content;
// getter and setter methods
}
```
接下来,创建一个数据访问层的接口,继承自JpaRepository接口:
```java
public interface CmsContentRepository extends JpaRepository<CmsContent, Long> {
}
```
然后,创建一个控制器类来处理HTTP请求:
```java
@RestController
@RequestMapping("/cms")
public class CmsController {
@Autowired
private CmsContentRepository cmsContentRepository;
@GetMapping
public List<CmsContent> getAllCmsContent() {
return cmsContentRepository.findAll();
}
@PostMapping
public CmsContent createCmsContent(@RequestBody CmsContent cmsContent) {
return cmsContentRepository.save(cmsContent);
}
@PutMapping("/{id}")
public CmsContent updateCmsContent(@PathVariable Long id, @RequestBody CmsContent updatedCmsContent) {
CmsContent cmsContent = cmsContentRepository.findById(id)
.orElseThrow(() -> new ResourceNotFoundException("CmsContent", "id", id));
cmsContent.setTitle(updatedCmsContent.getTitle());
cmsContent.setContent(updatedCmsContent.getContent());
return cmsContentRepository.save(cmsContent);
}
@DeleteMapping("/{id}")
public ResponseEntity<?> deleteCmsContent(@PathVariable Long id) {
CmsContent cmsContent = cmsContentRepository.findById(id)
.orElseThrow(() -> new ResourceNotFoundException("CmsContent", "id", id));
cmsContentRepository.delete(cmsContent);
return ResponseEntity.ok().build();
}
}
```
最后,创建一个HTML页面来展示CMS内容列表和表单:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>CMS Content Management</title>
<link rel="stylesheet" href="/webjars/element-ui/2.15.1/theme-chalk/index.css">
</head>
<body>
<div id="app">
<el-table :data="cmsContentList" style="width: 100%">
<el-table-column prop="title" label="Title"></el-table-column>
<el-table-column prop="content" label="Content"></el-table-column>
<el-table-column label="Actions">
<template slot-scope="scope">
<el-button type="danger" @click="deleteCmsContent(scope.row.id)">Delete</el-button>
</template>
</el-table-column>
</el-table>
<el-form :model="cmsContentForm" label-width="80px">
<el-form-item label="Title">
<el-input v-model="cmsContentForm.title"></el-input>
</el-form-item>
<el-form-item label="Content">
<el-input v-model="cmsContentForm.content"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="createCmsContent">Save</el-button>
</el-form-item>
</el-form>
</div>
<script src="/webjars/element-ui/2.15.1/index.js"></script>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
new Vue({
el: '#app',
data: {
cmsContentList: [],
cmsContentForm: {
title: '',
content: ''
}
},
methods: {
getCmsContentList() {
axios.get('/cms')
.then(response => {
this.cmsContentList = response.data;
})
.catch(error => {
console.error(error);
});
},
createCmsContent() {
axios.post('/cms', this.cmsContentForm)
.then(response => {
this.cmsContentList.push(response.data);
this.cmsContentForm.title = '';
this.cmsContentForm.content = '';
})
.catch(error => {
console.error(error);
});
},
deleteCmsContent(id) {
axios.delete(`/cms/${id}`)
.then(() => {
this.cmsContentList = this.cmsContentList.filter(cmsContent => cmsContent.id !== id);
})
.catch(error => {
console.error(error);
});
}
},
mounted() {
this.getCmsContentList();
}
});
</script>
</body>
</html>
```
这只是一个简单的示例,你可以根据自己的需求进行扩展和定制。希望对你有所帮助!
相关推荐
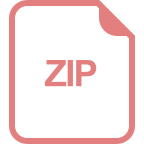
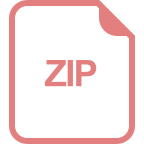
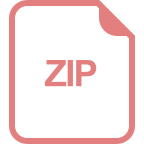














