银行家算法实验java
时间: 2023-12-19 11:31:59 浏览: 65
以下是一个简单的Java实现银行家算法的示例代码:
```java
import java.util.Scanner;
public class BankerAlgorithm {
private int[][] max; // 最大需求矩阵
private int[][] allocation; // 已分配矩阵
private int[][] need; // 需求矩阵
private int[] available; // 可用资源向量
private int processNum; // 进程数
private int resourceNum; // 资源种类数
public BankerAlgorithm(int[][] max, int[][] allocation, int[] available) {
this.max = max;
this.allocation = allocation;
this.available = available;
this.processNum = max.length;
this.resourceNum = available.length;
this.need = new int[processNum][resourceNum];
for (int i = 0; i < processNum; i++) {
for (int j = 0; j < resourceNum; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
}
public boolean isSafe() {
boolean[] finish = new boolean[processNum];
int[] work = new int[resourceNum];
for (int i = 0; i < resourceNum; i++) {
work[i] = available[i];
}
int count = 0;
while (count < processNum) {
boolean flag = false;
for (int i = 0; i < processNum; i++) {
if (!finish[i]) {
boolean temp = true;
for (int j = 0; j < resourceNum; j++) {
if (need[i][j] > work[j]) {
temp = false;
break;
}
}
if (temp) {
for (int j = 0; j < resourceNum; j++) {
work[j] += allocation[i][j];
}
finish[i] = true;
flag = true;
count++;
}
}
}
if (!flag) {
return false;
}
}
return true;
}
public boolean requestResource(int pid, int[] request) {
for (int i = 0; i < resourceNum; i++) {
if (request[i] > need[pid][i] || request[i] > available[i]) {
return false;
}
}
for (int i = 0; i < resourceNum; i++) {
available[i] -= request[i];
allocation[pid][i] += request[i];
need[pid][i] -= request[i];
}
return true;
}
public void releaseResource(int pid, int[] release) {
for (int i = 0; i < resourceNum; i++) {
available[i] += release[i];
allocation[pid][i] -= release[i];
need[pid][i] += release[i];
}
}
public static void main(String[] args) {
int[][] max = {{7, 5, 3}, {3, 2, 2}, {9, 0, 2}, {2, 2, 2}, {4, 3, 3}};
int[][] allocation = {{0, 1, 0}, {2, 0, 0}, {3, 0, 2}, {2, 1, 1}, {0, 0, 2}};
int[] available = {3, 3, 2};
BankerAlgorithm bankerAlgorithm = new BankerAlgorithm(max, allocation, available);
if (bankerAlgorithm.isSafe()) {
System.out.println("当前状态安全!");
} else {
System.out.println("当前状态不安全!");
}
Scanner scanner = new Scanner(System.in);
System.out.print("请输入请求资源的进程编号:");
int pid = scanner.nextInt();
System.out.print("请输入请求的资源向量:");
int[] request = new int[bankerAlgorithm.resourceNum];
for (int i = 0; i < bankerAlgorithm.resourceNum; i++) {
request[i] = scanner.nextInt();
}
if (bankerAlgorithm.requestResource(pid, request)) {
if (bankerAlgorithm.isSafe()) {
System.out.println("当前状态安全!");
} else {
System.out.println("当前状态不安全!");
bankerAlgorithm.releaseResource(pid, request);
}
} else {
System.out.println("请求被拒绝!");
}
}
}
```
阅读全文
相关推荐

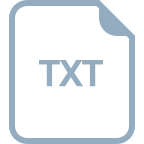
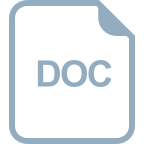
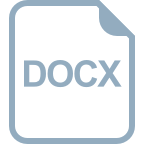
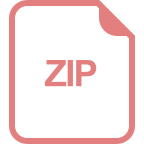
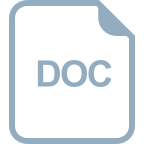
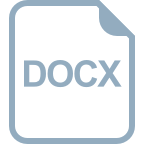
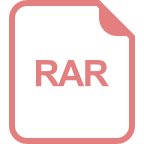
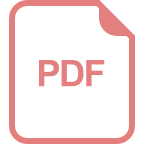
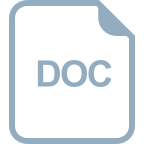
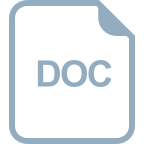
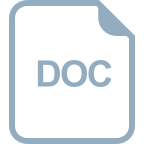