图片转成base64 python
时间: 2024-06-18 10:01:36 浏览: 17
在Python中,将图片转换为Base64编码是一个常见的操作,特别是当你需要在网络上传输图片数据但又不想保存文件时。以下是一个简单的步骤来实现这个功能:
```python
import io
import base64
def image_to_base64(image_path):
# 读取图片文件
with open(image_path, 'rb') as img_file:
# 使用BytesIO处理二进制数据
img_byte_arr = io.BytesIO(img_file.read())
# 转换为Base64编码
base64_str = base64.b64encode(img_byte_arr.getvalue()).decode('utf-8')
return base64_str
# 使用方法
image_path = 'path_to_your_image.jpg'
encoded_image = image_to_base64(image_path)
print("Base64 Encoded Image: ", encoded_image)
相关问题
图片转base64 python
在Python中,可以使用Pillow库来进行图片处理和转换。具体的步骤如下:
1. 安装Pillow库:可以使用pip命令来进行安装,如下所示:
```
pip install Pillow
```
2. 加载图片:使用Image.open()方法来加载图片,如下所示:
```
from PIL import Image
img = Image.open("image.jpg")
```
3. 将图片转换为base64编码:使用base64模块的b64encode()方法来将图片数据转换为base64编码,如下所示:
```
import base64
with open("image.jpg", "rb") as image_file:
encoded_string = base64.b64encode(image_file.read())
```
其中,"image.jpg"为待转换的图片文件名。
python numpy图片转base64
在Python中,可以使用numpy和base64库来实现将图片转换为base64编码的操作。下面是一个示例代码:
```python
import numpy as np
import base64
def image_to_base64(image_path):
# 读取图片并转换为numpy数组
image = np.array(Image.open(image_path))
# 将numpy数组转换为base64编码
image_base64 = base64.b64encode(image).decode('utf-8')
return image_base64
```
在上面的代码中,首先使用numpy库将图片读取为一个numpy数组。然后,使用base64库的b64encode函数将numpy数组转换为base64编码。最后,使用decode('utf-8')将编码转换为字符串格式。
你可以调用image_to_base64函数,并传入图片路径作为参数,即可得到图片的base64编码。
相关推荐
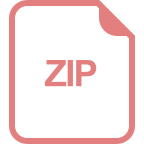












