分别使用3种方式创建学生对象,定义姓名、年龄、性别等属性,打招呼和跑的方法,使用for in 来遍历对象,将对象的属性和方法输出
时间: 2024-09-30 17:09:31 浏览: 46
当然可以。以下是三种创建学生对象的方式:
1. **传统JavaScript类**:
```javascript
class Student {
constructor(name, age, gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
sayHello() {
return `你好,我是${this.name},今年${this.age}岁。`;
}
run() {
return `${this.name}正在跑步...`;
}
}
// 创建3个实例
let student1 = new Student("小明", 18, "男");
let student2 = new Student("小红", 20, "女");
let student3 = new Student("小华", 19, "男");
// 使用for...in遍历并打印属性和方法
for (let attr in students = [student1, student2, student3]) {
console.log(`${attr}:`, students[attr]);
if (typeof students[attr] === 'function') {
console.log(`${attr}() => ${students[attr].call(students[attr])}`);
}
}
```
2. **ES6 JavaScript类(箭头函数)**:
```javascript
const Student = ({name, age, gender}) => ({
sayHello: () => `你好,我是${name},今年${age}岁.`,
run: () => `${name}正在跑步...`,
});
let student1 = Student({name: "小明", age: 18, gender: "男"});
let student2 = Student({name: "小红", age: 20, gender: "女"});
let student3 = Student({name: "小华", age: 19, gender: "男"});
// 遍历对象
for (let student of [student1, student2, student3]) {
for (let key in student) {
console.log(`${key}:`, student[key]);
if (typeof student[key] === 'function') {
console.log(`${key}() => ${student[key]()}`);
}
}
}
```
3. **使用JavaScript的对象字面量和原型链(模拟类)**:
```javascript
function Student(name, age, gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
Student.prototype.sayHello = function() {
return `你好,我是${this.name},今年${this.age}岁。`;
};
Student.prototype.run = function() {
return `${this.name}正在跑步...`;
};
// 创建3个实例
let student1 = new Student("小明", 18, "男");
let student2 = new Student("小红", 20, "女");
let student3 = new Student("小华", 19, "男");
// 遍历对象
for (let i = 0; i < 3; i++) {
let student = students[i];
for (let prop in student) {
console.log(`${prop}:`, student[prop]);
if (typeof student[prop] === 'function') {
console.log(`${prop}() => ${student[prop].call(student)}`);
}
}
}
```
阅读全文
相关推荐
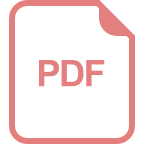
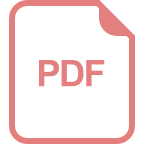
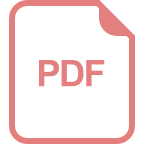
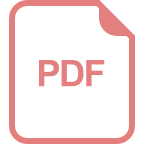
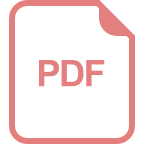
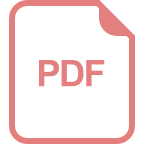
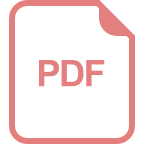
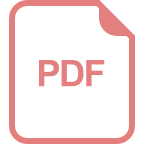
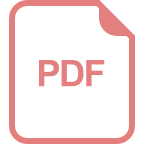
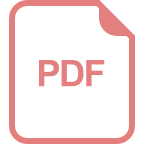
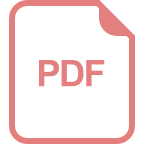
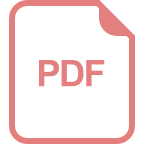
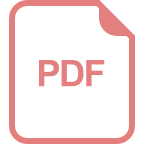
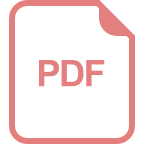
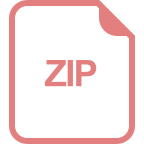
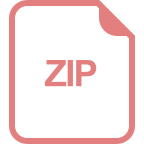
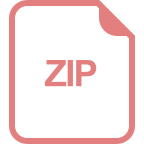
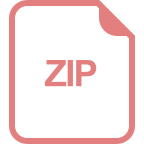