邻接矩阵和邻接表表示图java代码实现
时间: 2023-10-18 16:25:45 浏览: 56
邻接矩阵和邻接表是两种常用的图的表示方法。下面是Java代码实现邻接矩阵和邻接表的示例:
1. 邻接矩阵表示图的Java代码实现:
```java
import java.util.Arrays;
class Graph {
private int[][] adjMatrix;
private int numVertices;
public Graph(int numVertices) {
this.numVertices = numVertices;
this.adjMatrix = new int[numVertices][numVertices];
}
public void addEdge(int src, int dest) {
// 无向图,所以需要在两个位置都设置为1
adjMatrix[src][dest] = 1;
adjMatrix[dest][src] = 1;
}
public void printGraph() {
for (int i = 0; i < numVertices; i++) {
System.out.print("Vertex " + i + " is connected to: ");
for (int j = 0; j < numVertices; j++) {
if (adjMatrix[i][j] == 1) {
System.out.print(j + " ");
}
}
System.out.println();
}
}
}
public class Main {
public static void main(String[] args) {
Graph graph = new Graph(5);
graph.addEdge(0, 1);
graph.addEdge(0, 4);
graph.addEdge(1, 2);
graph.addEdge(1, 3);
graph.addEdge(1, 4);
graph.addEdge(2, 3);
graph.addEdge(3, 4);
graph.printGraph();
}
}
```
2. 邻接表表示图的Java代码实现:
```java
import java.util.LinkedList;
class Graph {
private int numVertices;
private LinkedList<Integer>[] adjList;
public Graph(int numVertices) {
this.numVertices = numVertices;
this.adjList = new LinkedList[numVertices];
for (int i = 0; i < numVertices; i++) {
adjList[i] = new LinkedList<>();
}
}
public void addEdge(int src, int dest) {
adjList[src].add(dest);
adjList[dest].add(src);
}
public void printGraph() {
for (int i = 0; i < numVertices; i++) {
System.out.print("Vertex " + i + " is connected to: ");
for (int j : adjList[i]) {
System.out.print(j + " ");
}
System.out.println();
}
}
}
public class Main {
public static void main(String[] args) {
Graph graph = new Graph(5);
graph.addEdge(0, 1);
graph.addEdge(0, 4);
graph.addEdge(1, 2);
graph.addEdge(1, 3);
graph.addEdge(1, 4);
graph.addEdge(2, 3);
graph.addEdge(3, 4);
graph.printGraph();
}
}
```
以上代码示例分别实现了邻接矩阵和邻接表表示图的Java代码。你可以根据自己的需求进行修改和扩展。
相关推荐
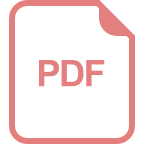
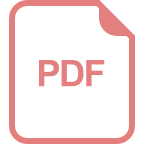
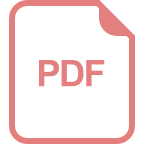














