用c语言设计一个线性表,采用链式储存结构,删除一个结点
时间: 2024-10-19 13:07:12 浏览: 44
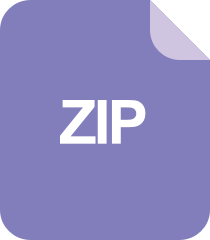
c语言数据结构实验:掌握线性表的链式存储结构 熟练掌握循环链表的存储特征和建立方法,掌握线性表的链式存储结构 下面是源码的txt
在 C 语言中,我们可以设计一个简单的单向链表数据结构,其中包含节点结构体,用于存储数据和指向下一个节点的指针。以下是一个基本的链表结构和删除节点的函数示例:
```c
// 定义链表节点结构体
typedef struct Node {
int data; // 节点的数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建一个新的节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 删除指定值的节点(如果存在)
void deleteNode(Node** head, int value) {
if (*head == NULL) {
printf("List is empty.\n");
return;
}
// 遍历链表寻找要删除的节点
Node* current = *head;
Node* previous = NULL;
while (current != NULL && current->data != value) {
previous = current;
current = current->next;
}
// 如果找到了节点
if (current != NULL) {
// 如果当前节点不是头节点
if (previous != NULL) {
previous->next = current->next;
} else { // 如果头节点就是要删除的
*head = current->next;
}
// 释放内存
free(current);
} else {
printf("Value not found in the list.\n");
}
}
// 添加节点到链表
void appendNode(Node** head, int value) {
Node* newNode = createNode(value);
if (newNode != NULL) {
if (*head == NULL) {
*head = newNode;
} else {
Node* last = *head;
while (last->next != NULL) {
last = last->next;
}
last->next = newNode;
}
}
}
// 打印链表
void printList(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
} else {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
}
int main() {
Node* head = NULL;
// 初始化和添加一些节点
appendNode(&head, 1);
appendNode(&head, 2);
appendNode(&head, 3);
printList(head); // 输出: 1 -> 2 -> 3 -> NULL
// 删除节点
deleteNode(&head, 2);
printList(head); // 输出: 1 -> 3 -> NULL
return 0;
}
```
这个程序展示了如何创建、插入、打印和删除链表节点的基本操作。`deleteNode` 函数会在找到匹配值的节点时将其从链表中移除。注意,在实际应用中,为了处理空链表和其他边界条件,还需要在适当的地方添加错误检查和处理代码。
阅读全文
相关推荐
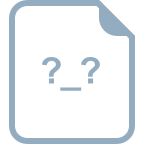
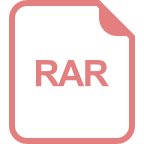















