在wpf中,bitmapImage转换为bitmap
时间: 2024-12-22 14:33:34 浏览: 12
在WPF中,如果你已经有一个`BitmapImage`对象,想要将其转换为`Bitmap`对象,可以直接使用`.BitmapSource`属性获取原始位图数据,然后再将其转换为`System.Drawing.Bitmap`。以下是这个过程的一个示例:
```csharp
using System.IO;
using System.Windows.Media.Imaging;
using System.Drawing;
// 假设你已经有了一个BitmapImage对象
BitmapImage bitmapImage = ...;
// 获取位图源数据
MemoryStream memoryStream = new MemoryStream();
bitmapImage.SaveJpeg(memoryStream, bitmapImage.Width, bitmapImage.Height);
memoryStream.Seek(0, SeekOrigin.Begin);
// 将内存流转换为System.Drawing.Bitmap
byte[] bitmapBytes = memoryStream.ToArray();
Bitmap bitmapFromBitmapImage = new Bitmap(bitmapBytes);
```
这里我们首先将`BitmapImage`保存为JPEG格式到内存流中,然后从内存流中读取字节数组,并用这些字节创建一个新的`System.Drawing.Bitmap`。
相关问题
在wpf中,bitmapImage转换为bitmap完整代码
在WPF中,你可以通过`WriteableBitmap`将`BitmapImage`转换为`Bitmap`。以下是完整的代码示例:
```csharp
using System.Windows.Media.Imaging;
using System.Drawing;
// 假设你已经有一个BitmapImage对象叫做bitmapImage
// 创建一个WriteableBitmap
var bitmap = new WriteableBitmap(bitmapImage.Width, bitmapImage.Height);
// 将BitmapImage的内容复制到WriteableBitmap
using (var stream = bitmapImage.GetStreamAt(0))
{
using (var graphicsContext = Graphics.FromImage(bitmap))
{
graphicsContext.DrawImage(bitmapImage, new Rectangle(0, 0, bitmapSource.PixelWidth, bitmapSource.PixelHeight));
}
}
// 现在你可以操作这个WriteableBitmap,就像普通的Bitmap一样
```
注意,`WriteableBitmap`允许你在内存中对位图进行直接绘制或修改,而`BitmapImage`则主要用于加载和显示图像资源。如果你需要保存为文件或其他用途,`WriteableBitmap`可以方便地导出为其他格式。
wpf把字节数据转换成bitmap
在WPF(Windows Presentation Foundation)中,将字节数据转换为Bitmap通常涉及以下几个步骤:
1. 获取字节数据:首先,你需要有一个包含图像字节的数据源,这可以是从文件、网络请求或程序内存中读取得到的。
2. 创建MemoryStream:创建一个`MemoryStream`,并将字节数据写入其中,因为Bitmap需要从流读取数据。
```csharp
MemoryStream byteStream = new MemoryStream();
byteStream.Write(byteData, 0, byteData.Length);
```
3. 初始化BitmapSource:利用`BitmapCreateFromStream`方法,创建一个`BitmapImage`对象,它是一个可以在WPF中使用的位图数据源。
```csharp
BitmapImage bitmapImage = new BitmapImage();
bitmapImage.BeginInit();
bitmapImage.StreamSource = byteStream;
bitmapImage.EndInit();
```
4. 设置或显示Bitmap:现在你可以将这个`BitmapImage`实例设置到UI元素的`Image`控件上,或者用于绘制等操作。
```csharp
imageView.Source = bitmapImage;
```
阅读全文
相关推荐
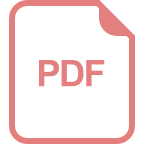
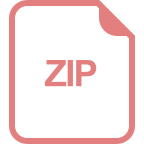
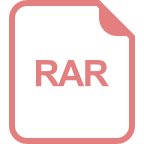

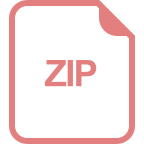
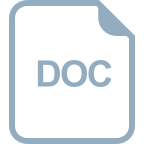
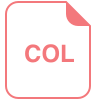









