不使用lsndfile实现解压缩opus文件成wav文件
时间: 2024-04-12 07:34:09 浏览: 189
您可以使用libopus库来解压缩.opus文件并将其转换为.wav文件,而无需使用libsndfile库。以下是一个简单示例代码,演示如何执行此操作:
```c
#include <opus/opus.h>
#include <stdio.h>
int main()
{
const char* input_filename = "input.opus";
const char* output_filename = "output.wav";
// 打开.opus文件
FILE* input_file = fopen(input_filename, "rb");
if (!input_file)
{
printf("无法打开输入文件\n");
return 1;
}
// 创建Opus解码器
int error;
OpusDecoder* decoder = opus_decoder_create(48000, 2, &error);
if (error != OPUS_OK)
{
printf("无法创建Opus解码器: %s\n", opus_strerror(error));
fclose(input_file);
return 1;
}
// 创建输出.wav文件
FILE* output_file = fopen(output_filename, "wb");
if (!output_file)
{
printf("无法创建输出文件\n");
opus_decoder_destroy(decoder);
fclose(input_file);
return 1;
}
// 解压缩并写入.wav文件
const int MAX_FRAME_SIZE = 960 * 6; // 最大帧大小为960样本(对于48kHz采样率和2个声道)
short output[MAX_FRAME_SIZE];
unsigned char input[MAX_FRAME_SIZE];
while (1)
{
// 从.opus文件中读取数据
size_t bytes_read = fread(input, sizeof(unsigned char), MAX_FRAME_SIZE, input_file);
if (bytes_read <= 0)
break;
// 解压缩数据
int frame_size = opus_decode(decoder, input, bytes_read, output, MAX_FRAME_SIZE, 0);
// 将解压缩的数据写入.wav文件
fwrite(output, sizeof(short), frame_size * 2, output_file);
}
// 关闭文件和解码器
fclose(input_file);
fclose(output_file);
opus_decoder_destroy(decoder);
printf("解压缩完成\n");
return 0;
}
```
请注意,您需要先安装libopus库,并使用正确的编译选项将其链接到您的项目中。此外,您还需要处理错误和异常情况以确保代码的健壮性。
阅读全文
相关推荐
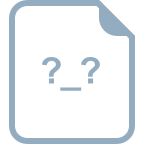
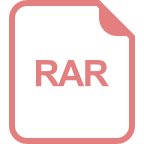
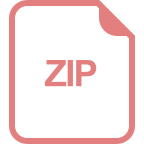

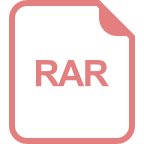
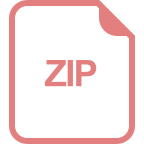
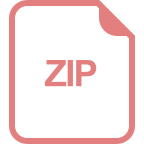
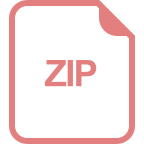
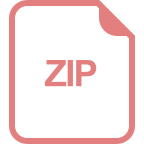
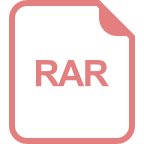
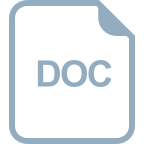






