springboot mybatisplus 创建表
时间: 2024-06-07 22:04:52 浏览: 16
Spring Boot 和 MyBatisPlus 是两个非常流行的技术组合,它们分别简化了Spring应用的初始设置和数据库操作。在Spring Boot项目中使用MyBatisPlus,你可以方便地进行CRUD操作,而无需手动编写大量的SQL语句。
创建表的过程主要包含以下几个步骤:
1. 添加依赖:首先,在你的Spring Boot项目的pom.xml或build.gradle文件中添加MyBatisPlus的依赖。例如,对于Maven项目:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.x.x</version> <!-- 根据实际版本号替换 -->
</dependency>
```
对于Gradle项目:
```groovy
implementation 'com.baomidou:mybatis-plus-boot-starter:3.x.x' // 替换为实际版本号
```
2. 配置mybatis-plus:在application.properties或application.yml中配置数据源和mybatis plus的全局配置,如:
```properties
spring.datasource.type=com.alibaba.druid.DruidDataSource
mybatis-plus.global-config={dbType=MySQL, tableNamePrefix="your_table_prefix_"} // 表名前缀
```
3. 创建实体类(Entity):定义Java对象,它将映射到数据库中的表,使用@Table注解指定表名:
```java
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.TableName;
import java.time.LocalDateTime;
@TableName("your_table_name")
public class YourEntity {
@TableId(value = "id", type = IdType.AUTO) // 主键自增
private Long id;
private String name;
@TableField(fill = FieldFill.LAZY) // 控制字段是否填充
private LocalDateTime createdAt;
// 其他字段...
}
```
4. 使用Mapper接口:MyBatisPlus提供了基于接口的编码方式,不需要写XML映射文件。在对应的包下创建Mapper接口:
```java
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.example.YourEntity;
public interface YourEntityMapper extends BaseMapper<YourEntity> {
}
```
5. 注册Mapper:在@Service或@Repository中注入Mapper,并注册到Spring容器中:
```java
@Service
public class YourService {
private final YourEntityMapper yourEntityMapper;
@Autowired
public YourService(YourEntityMapper yourEntityMapper) {
this.yourEntityMapper = yourEntityMapper;
}
public void createTable() {
yourEntityMapper.createTable(); // 自动生成表结构
}
}
```
相关推荐
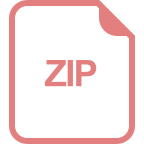














