int insert mybatis
时间: 2023-10-22 15:02:56 浏览: 97
To insert data using MyBatis, you need to follow these steps:
1. Define a mapper interface: Create an interface that defines the SQL statements to insert data. Annotate the method with `@Insert` and provide the SQL query.
```java
public interface YourMapper {
@Insert("INSERT INTO your_table (column1, column2) VALUES (#{value1}, #{value2})")
void insertData(@Param("value1") String value1, @Param("value2") String value2);
}
```
2. Configure the mapper: In the MyBatis configuration file (usually named `mybatis-config.xml`), add the mapper interface as a mapper element.
```xml
<mappers>
<mapper resource="com/example/YourMapper.xml" />
</mappers>
```
3. Execute the insert statement: Obtain an instance of `SqlSession` and use it to execute the insert statement defined in the mapper interface.
```java
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
SqlSession sqlSession = sqlSessionFactory.openSession();
YourMapper mapper = sqlSession.getMapper(YourMapper.class);
mapper.insertData("value1", "value2");
sqlSession.commit();
sqlSession.close();
```
Make sure to replace `your_table`, `column1`, `column2`, and the values with your own table and column names.
Note: This example assumes you have already set up MyBatis and have the necessary dependencies in your project.
阅读全文
相关推荐
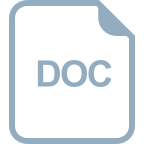
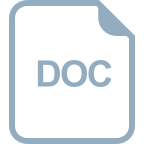
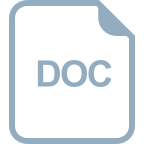
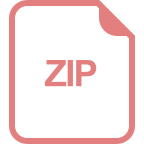














