while(!my_File.eof()) { char buffer[100] = {0}; if(my_File.getline(line, sizeof(line))) { while(my_File >> Sort_Code >> Link_ID >> Turn_Road >> Name_Count >> Road_Name) { cout <<"分类编码:"<< Sort_Code << endl; cout << "链接ID:" << Link_ID << endl; cout << "分叉路口数:"<< Turn_Road << endl; cout << "名字数量:" << Name_Count << endl; cout << "道路名称:" << Road_Name << endl << endl; } } //else if (getchar() == '\n') continue; }这行代码是什么意思
时间: 2023-08-20 14:01:12 浏览: 44
这段代码是一个文件读取的循环。它的意思是,在文件未结束之前,不断执行循环内的代码。
在循环内部,首先定义了一个名为`buffer`的字符数组,并将其初始化为全零。然后使用`getline`函数从文件中读取一行内容,并将其存储在`line`字符数组中。接下来,使用输入流运算符`>>`从文件中依次读取`Sort_Code`、`Link_ID`、`Turn_Road`、`Name_Count`和`Road_Name`的值,并将它们输出到控制台上。
然后,程序会继续读取下一行的内容,并重复执行上述操作,直到文件结束。
注释部分代码 `//else if (getchar() == '\n') continue;` 是一个被注释掉的条件判断语句,它的作用是在遇到换行符时跳过当前循环,继续下一次循环。
相关问题
while(!my_File.eof()) { char buffer[100] = {0}; if(my_File.getline(line, sizeof(line))) { while(my_File >> Sort_Code >> Link_ID >> Turn_Road >> Name_Count >> Road_Name) { cout <<"分类编码:"<< Sort_Code << endl; cout << "链接ID:" << Link_ID << endl; cout << "分叉路口数:"<< Turn_Road << endl; cout << "名字数量:" << Name_Count << endl; cout << "道路名称:" << Road_Name << endl << endl; } } //else if (getchar() == '\n') continue; }这段代码是否有错
这段代码看起来没有明显的语法错误。然而,需要注意一些潜在的问题。
1. `my_File.eof()`:使用`eof()`函数来判断文件是否已经到达末尾是不可靠的,因为在读取完最后一行之后,`eof()`函数可能还不会返回`true`。建议使用其他方式来判断文件是否结束,比如在读取失败后检查`failbit`标志位。
2. `getline(line, sizeof(line))`:这里假设`line`是一个字符数组,但是代码中没有给出`line`的定义。确保在使用之前,`line`被正确定义和初始化。
3. 循环内部的读取操作:在每次循环中,使用输入流运算符`>>`从文件中读取数据。如果读取失败(比如遇到文件结束或者文件格式错误),循环可能会陷入无限循环。建议在读取操作后检查流状态,以确保读取成功。
除了以上提到的问题,这段代码的逻辑可能需要根据实际需求进行调整和优化。
#include<iostream> #include<fstream> using namespace std; int main() { fstream in; char buffer[100]; in.open("12.cpp",ios::in); fstream out("12.txt",ios::out); int line_nume=0; while(!in.eof())//若读到文件尾部才结束 { in.getline(buffer,100); ++line_nume; out<<line_nume<<":"<<buffer<<endl; cout<<line_nume<<":"<<buffer<<endl;//输出所读的文件内容 } in.close(); out.close(); return 0; } 优化这段代码
这段代码可以进行以下优化:
1. 使用 const string 定义文件名,避免在多个地方使用字符串字面量,可读性更好。
2. 使用 std::string 类型代替字符数组 buffer,更加安全。
3. 在 while 循环中使用 getline(),避免出现读取行数不对的情况。
4. 将文件打开和关闭的操作移到 while 循环外部,避免重复操作,提高效率。
5. 使用 ++line_num 代替 line_num++,提高效率。
6. 增加错误处理,比如文件打开失败后应该进行错误提示。
改进后的代码如下:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
const string FILE_NAME = "12.cpp";
int main() {
fstream in;
string buffer;
in.open(FILE_NAME, ios::in);
if (!in.is_open()) {
cerr << "Failed to open file: " << FILE_NAME << endl;
return 1;
}
fstream out("12.txt", ios::out);
int line_num = 0;
while (getline(in, buffer)) {
++line_num;
out << line_num << ":" << buffer << endl;
cout << line_num << ":" << buffer << endl;
}
in.close();
out.close();
return 0;
}
```
相关推荐








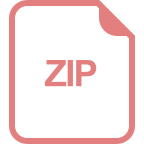
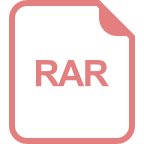
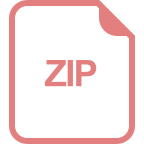
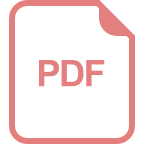