stream循环里做加法运算
时间: 2024-09-25 15:15:25 浏览: 26
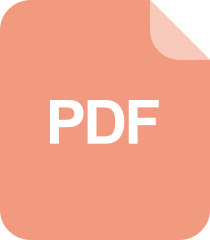
Stream安装配置教程[总结].pdf
在Java Stream API中,如果你想在流(Stream)循环中做加法运算,通常我们会将所有元素转换成数值类型,然后使用`reduce()`函数来累加。`reduce()`方法接受一个初始值和一个二元操作,可以将整个流中的元素按某种规则汇总。例如,如果你有一个整数流,你可以这样做:
```java
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.mapToInt(Integer::intValue) // 将Stream转为IntStream便于加法操作
.reduce(0, (a, b) -> a + b); // 使用reduce计算累加和,初始值设为0
System.out.println(sum); // 输出: 15
```
这里我们首先使用`mapToInt()`将每个Integer元素转换为Integers,然后传入`reduce()`函数,其中`(a, b) -> a + b`是一个lambda表达式,它表示每次迭代都将当前累计值(a)加上下一个元素(b)。
阅读全文
相关推荐
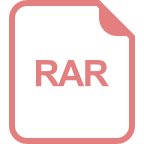
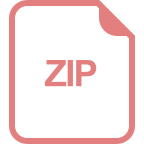
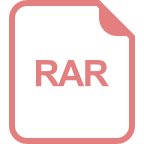
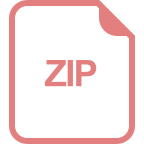
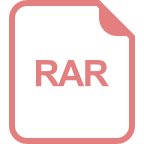
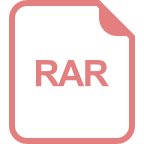
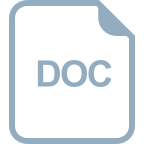
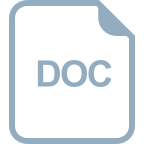
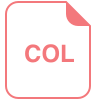
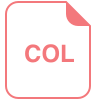
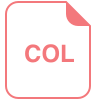



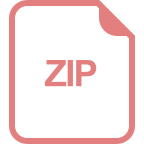
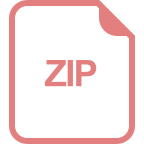
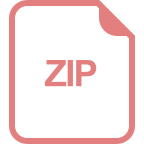