使用c++语言建立一个链式表,实现以下功能 1.设计C++类及相关方法,用于维护单链表 2.写出建立单链表,并向单链表中输入数据的函数。 3. 实现单链表建立、遍历、查找、新元素插入、及元素删除,写出输入及输出的内容。
时间: 2024-09-23 07:02:36 浏览: 26
在C++中,我们可以创建一个名为`LinkedList`的类来实现单链表的功能。这里我会提供一个简单的实现,包括节点类(`Node`)以及链表类(`LinkedList`)。首先,我们需要定义节点结构体:
```cpp
// 节点类
class Node {
public:
int data;
Node* next;
// 构造函数
Node(int value) : data(value), next(nullptr) {}
};
```
接下来,我们定义链表类及其操作方法:
```cpp
// 链表类
class LinkedList {
private:
Node* head; // 指向链表头部的指针
public:
// 构造函数,初始化空链表
LinkedList() : head(nullptr) {}
// 添加元素到链表尾部
void add(int value) {
Node* newNode = new Node(value);
if (head == nullptr) {
head = newNode;
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
// 遍历并打印链表
void display() const {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << "\n";
}
// 查找元素
bool find(int value) const {
Node* current = head;
while (current != nullptr) {
if (current->data == value) {
return true;
}
current = current->next;
}
return false;
}
// 插入元素到指定位置
void insertAtPosition(int position, int value) {
if (position <= 0) {
std::cerr << "Invalid position.\n";
return;
}
Node* newNode = new Node(value);
if (position == 1) { // 如果要在头部插入
newNode->next = head;
head = newNode;
} else {
Node* current = head;
for (int i = 1; i < position - 1 && current != nullptr; ++i) {
current = current->next;
}
if (current != nullptr) {
newNode->next = current->next;
current->next = newNode;
} else {
std::cerr << "Position out of range.\n";
}
}
}
// 删除元素
void remove(int value) {
if (head == nullptr) {
return;
}
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
Node* previous = nullptr;
while (current != nullptr && current->data != value) {
previous = current;
current = current->next;
}
if (current != nullptr) {
previous->next = current->next;
delete current;
} else {
std::cerr << "Element not found in the list.\n";
}
}
};
// 建立链表并添加元素的函数
void createLinkedList(LinkedList& list, const std::vector<int>& values) {
for (const auto& value : values) {
list.add(value);
}
}
```
现在你可以用这些方法来创建、操作和显示链表了。例如,你可以这样做:
```cpp
int main() {
LinkedList myList;
std::vector<int> values = {1, 2, 3, 4, 5};
createLinkedList(myList, values);
// 输出初始链表
myList.display();
// 查找元素
if (myList.find(3)) {
std::cout << "Found element 3.\n";
} else {
std::cout << "Element 3 not found.\n";
}
// 在特定位置插入元素
myList.insertAtPosition(3, 6); // 在索引3处插入值6
myList.display();
// 删除元素
myList.remove(4);
myList.display();
return 0;
}
```
相关推荐
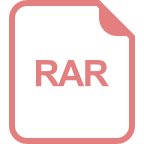
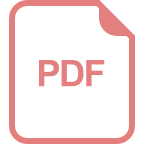
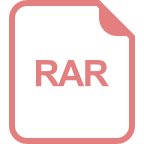

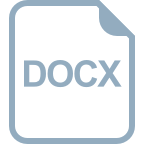
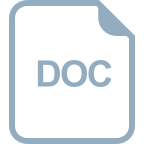
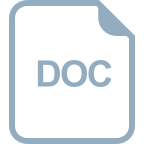
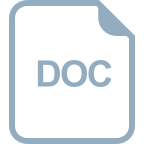
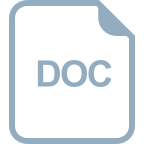
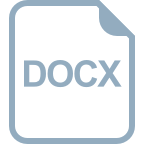
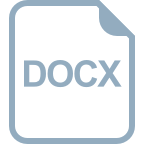
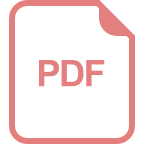
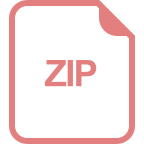
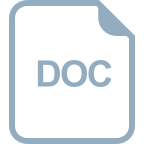
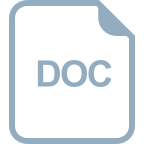
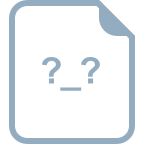
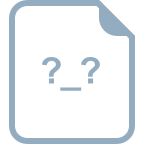