使用c++语言建立一个链式表,实现以下功能 1.设计C++类及相关方法,用于维护单链表 2.写出建立单链表,并向单链表中输入数据的函数。 3. 实现单链表建立、遍历、查找、新元素插入、及元素删除,写出输入及输出的内容。
时间: 2024-09-23 18:02:30 浏览: 24
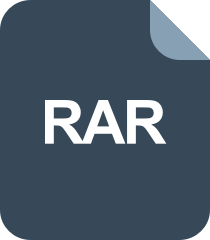
谭浩强C语言程序设计,C++程序设计,严蔚敏数据结构,高一凡数据结构算法分析与实现.rar )

在C++中,我们可以创建一个名为`ListNode`的链表节点类以及相关的链表类`LinkedList`来实现上述功能。以下是基本的设计:
1. `ListNode`类定义:
```cpp
class ListNode {
public:
int data;
ListNode* next;
// 构造函数
ListNode(int value) : data(value), next(nullptr) {}
};
```
2. `LinkedList`类定义:
```cpp
class LinkedList {
private:
ListNode* head; // 链表头节点
public:
LinkedList() : head(nullptr) {}
// 添加节点到链表末尾
void addNode(int value) {
ListNode* newNode = new ListNode(value);
if (!head) {
head = newNode;
} else {
ListNode* current = head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
}
// 遍历链表并打印节点值
void traverse() {
ListNode* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
// 查找特定值的节点
ListNode* search(int value) {
ListNode* current = head;
while (current != nullptr) {
if (current->data == value) {
return current;
}
current = current->next;
}
return nullptr; // 如果未找到,返回nullptr
}
// 在指定位置插入新节点
void insertAtPosition(int value, int position) {
if (position <= 0) {
throw std::invalid_argument("Invalid position");
}
ListNode* newNode = new ListNode(value);
if (position == 1) { // 插入在头部
newNode->next = head;
head = newNode;
} else {
ListNode* previous = head;
for (int i = 1; i < position - 1 && previous != nullptr; ++i) {
previous = previous->next;
}
if (previous == nullptr) {
throw std::runtime_error("Position out of range");
}
newNode->next = previous->next;
previous->next = newNode;
}
}
// 删除节点
bool removeNode(int value) {
if (head == nullptr) {
return false;
}
if (head->data == value) {
ListNode* temp = head;
head = head->next;
delete temp;
return true;
}
ListNode* current = head;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next == nullptr) {
return false;
}
ListNode* toDelete = current->next;
current->next = current->next->next;
delete toDelete;
return true;
}
};
// 示例
int main() {
LinkedList list;
list.addNode(1);
list.addNode(2);
list.addNode(3);
list.traverse(); // 输出:1 2 3
ListNode* found = list.search(2); // 找到节点2
if (found) {
std::cout << "Found node with value: " << found->data << std::endl;
} else {
std::cout << "Node not found" << std::endl;
}
list.insertAtPosition(4, 2); // 插入4在位置2
list.traverse(); // 输出:1 2 4 3
list.removeNode(2); // 删除值为2的节点
list.traverse(); // 输出:1 4 3
return 0;
}
```
阅读全文
相关推荐
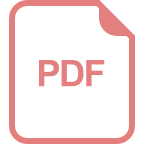
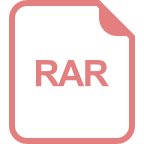

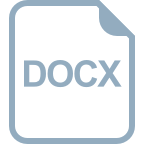
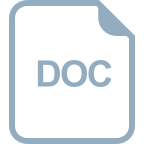
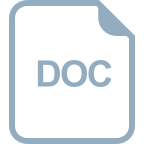
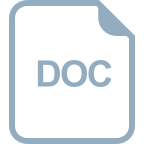
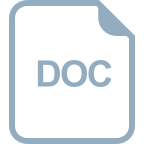
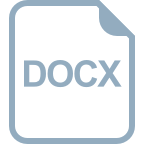
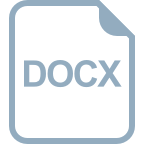
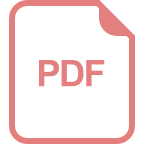
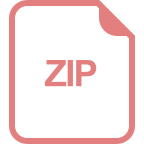
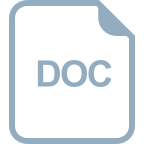
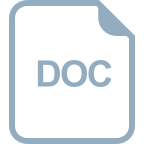
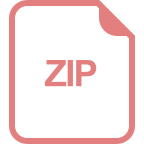
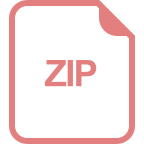
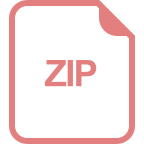