写一个ardiuno mega2560 代码 用于控制delta robot
时间: 2024-10-16 15:07:19 浏览: 49
Delta机器人是一种由三个臂组成的并联机器人,通常用于高精度定位。为了在Arduino Mega 2560上编写控制Delta机器人的代码,你需要了解一些基本概念,如电机控制、PID算法(如果需要精确位置控制)以及脉冲宽度调制(PWM)信号。下面是一个简单的示例代码,假设你有三个步进电机连接到Mega2560的数字引脚,并且每个电机驱动器都配备了限位传感器:
```cpp
#include <Stepper.h>
// 定义电机步进电机配置
const int stepPinA = 9; // 电机A的步骤信号
const int dirPinA = 8; // 电机A的方向信号
const int limitPinA = 7; // 电机A的限位信号
const int stepPinB = 10; // 电机B配置类似...
const int dirPinB = 11;
const int limitPinB = 12;
const int stepPinC = 5; // 电机C配置类似...
const int dirPinC = 4;
const int limitPinC = 3;
// 创建电机对象
Stepper motorA(stepPinA, dirPinA);
Stepper motorB(stepPinB, dirPinB);
Stepper motorC(stepPinC, dirPinC);
// PID控制器设置(假设已初始化)
double kp = 0.5; // 比例增益
double ki = 0.1; // 积分增益
double kd = 0.01; // 微分增益
double setpoint; // 目标位置
double error; // 当前误差
double integral; // 积分项
double derivative; // 微分项
long prevTime; // 上一次更新时间
void setup() {
Serial.begin(9600); // 开启串口通信
}
void loop() {
// 更新目标位置 (这里仅作为示例)
setpoint += 1; // 每次增加1度的角度
// 更新PID计算
long currentTime = millis();
double dt = currentTime - prevTime;
error = setpoint - getMotorPosition(); // 获取实际位置函数
integral += error * dt;
derivative = (error - prevError) / dt;
// 控制电机运动
motorA.runSpeed(kp * error + ki * integral + kd * derivative);
motorB.runSpeed(kp * error + ki * integral + kd * derivative);
motorC.runSpeed(kp * error + ki * integral + kd * derivative);
if (limitPinA == LOW || limitPinB == LOW || limitPinC == LOW) { // 判断到达极限
stopMotors();
Serial.println("Limit reached, resetting position.");
resetPosition(); // 自定义重置位置的函数
}
prevError = error;
prevTime = currentTime;
}
// 停止所有电机
void stopMotors() {
motorA.stop();
motorB.stop();
motorC.stop();
}
// 返回电机当前位置函数 (这里仅作示例,需要根据实际情况实现)
int getMotorPosition() {
return 0; // 需要你自己实现获取电机当前位置的方法
}
// 重置电机位置函数 (同样需要自定义实现)
void resetPosition() {
motorA.moveTo(0);
motorB.moveTo(0);
motorC.moveTo(0);
}
```
注意:这个代码是一个基础示例,实际应用中还需要考虑硬件连接、错误处理、速度控制等因素,并根据电机的具体型号和电路调整代码。此外,对于Delta机器人,可能还需要额外的校准步骤来确保准确的位置。
阅读全文
相关推荐
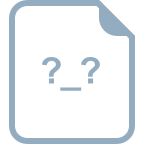
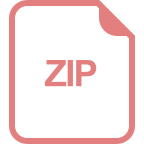
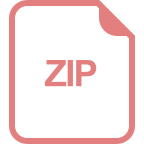
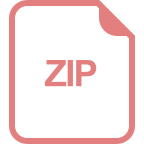
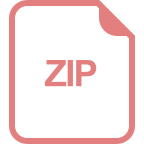
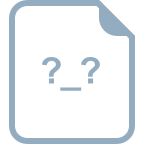
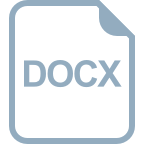
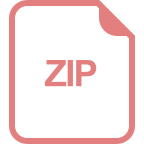
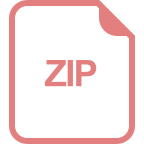
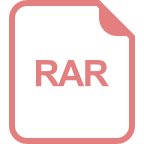
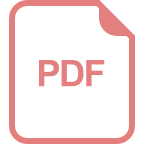
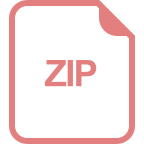
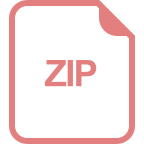
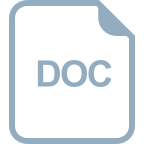