C语言-链表-添加到链表尾部
时间: 2024-01-18 15:04:03 浏览: 117
以下是C语言中将新节点添加到链表尾部的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
struct Node {
int data;
struct Node* next;
};
// 在链表尾部添加新节点
void append(struct Node** head_ref, int new_data) {
// 为新节点分配内存
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
struct Node* last = *head_ref; // 用于遍历链表
// 设置新节点的值和下一个节点为NULL
new_node->data = new_data;
new_node->next = NULL;
// 如果链表为空,则将新节点设置为头节点
if (*head_ref == NULL) {
*head_ref = new_node;
return;
}
// 遍历链表,找到最后一个节点
while (last->next != NULL) {
last = last->next;
}
// 将新节点添加到链表尾部
last->next = new_node;
return;
}
// 测试代码
int main() {
struct Node* head = NULL; // 初始化链表为空
// 添加节点
append(&head, 1);
append(&head, 2);
append(&head, 3);
// 遍历链表并输出每个节点的值
struct Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
return 0;
}
```
阅读全文
相关推荐
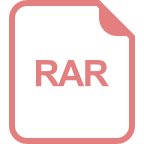



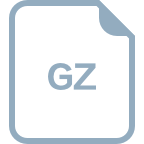




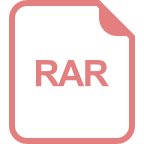





