c语言创建链表
时间: 2023-08-10 19:22:23 浏览: 108
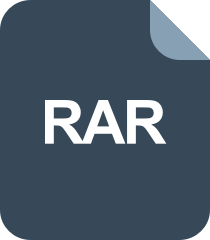
c语言实现链表的基本操作,链表是一种物理存储单元上非连续、非顺序的存储结构,数据元素的逻辑顺序是通过链表中的指针链接次序实现的。
创建链表的基本步骤如下:
1. 定义链表节点结构体
```c
struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
};
```
2. 创建链表头节点
```c
struct Node* head = NULL; // 初始为空链表
```
3. 创建新的节点并插入链表
```c
// 在链表尾部插入新节点
void insert(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
return;
}
struct Node* cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = newNode;
}
```
4. 遍历链表输出节点数据
```c
void printList() {
struct Node* cur = head;
while (cur != NULL) {
printf("%d ", cur->data);
cur = cur->next;
}
}
```
完整代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* head = NULL;
void insert(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
return;
}
struct Node* cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = newNode;
}
void printList() {
struct Node* cur = head;
while (cur != NULL) {
printf("%d ", cur->data);
cur = cur->next;
}
}
int main() {
insert(1);
insert(2);
insert(3);
printList();
return 0;
}
```
阅读全文
相关推荐
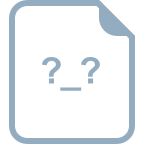
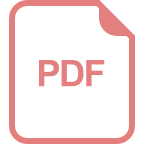
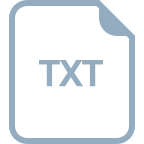
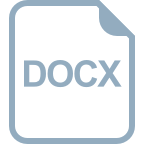
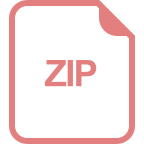
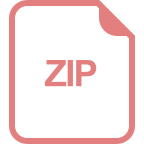
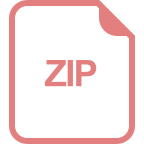
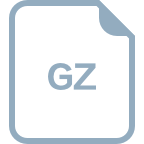
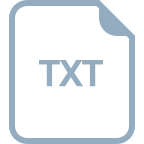
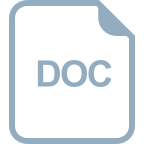
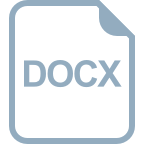
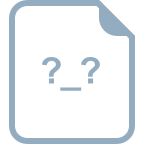
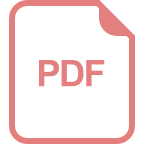
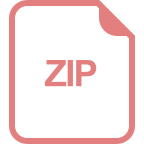
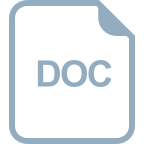
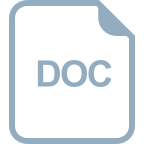