单片机流水灯代码字节控制
时间: 2024-10-14 16:08:12 浏览: 32
单片机流水灯是一种常见的电子小项目,通常使用微控制器如8051系列的芯片通过I/O口控制LED灯串逐个点亮。基本的代码实现会涉及到以下几个步骤:
1. 初始化:设置I/O口的工作模式为输出,并将所有LED初始化为高电平(关闭状态)。
```c
void init_lights() {
DDR某某 = 0xFF; // 设置IO口为输出
}
```
2. 循环操作:创建一个循环,通过位移操作控制LED灯的亮灭。比如,如果用P1口(假设它是8个LED)组成一串,可以先点亮第一个LED,然后将其置为低电平并点亮下一个LED,依次类推。
```c
void shift_lights() {
for (int i = 0; i < 8; ++i) {
P1 |= 1 << i; // 点亮当前LED
delay_ms(10); // 等待一段时间再切换
P1 &= ~(1 << i); // 关闭当前LED
}
}
```
3. 主循环:在一个无限循环中调用`init_lights()`初始化,然后不断轮询`shift_lights()`。
```c
while (1) {
init_lights();
shift_lights();
}
```
相关问题
52单片机流水灯代码
52单片机流水灯代码是一种基于C语言编写的程序,通过控制单片机的输出口实现流水灯效果。具体实现方法是利用循环左移和循环右移操作符,将一个字节的数据在8个LED灯之间循环移位,从而实现流水灯效果。以下是一个简单的52单片机流水灯代码示例:
#include<reg52.h>
#include<intrins.h>
#define uint unsigned int
#define uchar unsigned char
uchar temp;
void delay(uint z);
void main()
{
temp=0xfe; //初始值为11111110,即第一个灯亮,其余灯灭
P1=temp; //将初始值输出到P1口
while(1)
{
temp=_crol_(temp,1); //循环左移一位
delay(600); //延时一段时间
P1=temp; //将移位后的值输出到P1口
}
}
void delay(uint z)
{
uint x,y;
for(x=100;x>0;x--)
for(y=z;y>0;y--);
}
以上代码中,P1口为单片机的输出口,temp为一个字节的变量,用于存储流水灯的状态。在主函数中,先将temp的初始值设为11111110,即第一个灯亮,其余灯灭,然后进入一个无限循环中。在循环中,先将temp循环左移一位,然后延时一段时间,最后将移位后的值输出到P1口,从而实现流水灯效果。
stc15单片机流水灯代码
以下是STC15单片机流水灯的代码,使用了WS2812灯带:
```c
#include <reg52.h>
#include <intrins.h>
#define uchar unsigned char
#define uint unsigned int
sbit LED = P1^0;
// 定义WS2812灯带的数据格式
typedef struct {
uchar G;
uchar R;
uchar B;
} Color;
// 发送一个字节的数据到WS2812灯带
void sendByte(uchar dat) {
uchar i;
for (i = 0; i < 8; i++) {
if (dat & 0x80) {
LED = 1;
_nop_();
_nop_();
LED = 0;
} else {
LED = 1;
_nop_();
LED = 0;
_nop_();
}
dat <<= 1;
}
}
// 发送一个颜色数据到WS2812灯带
void sendColor(Color color) {
sendByte(color.G);
sendByte(color.R);
sendByte(color.B);
}
// 发送多个颜色数据到WS2812灯带
void sendColors(Color *colors, uint count) {
uint i;
for (i = 0; i < count; i++) {
sendColor(colors[i]);
}
}
// 流水灯效果
void flowLight(Color *colors, uint count, uint delay) {
uint i, j;
for (i = 0; i < count; i++) {
for (j = 0; j < count; j++) {
colors[j].R = 0;
colors[j].G = 0;
colors[j].B = 0;
}
colors[i].R = 255;
colors[i].G = 255;
colors[i].B = 255;
sendColors(colors, count);
delay_ms(delay);
}
}
void main() {
Color colors[12];
uint i;
for (i = 0; i < 12; i++) {
colors[i].R = 0;
colors[i].G = 0;
colors[i].B = 0;
}
while (1) {
flowLight(colors, 12, 100);
}
}
```
阅读全文
相关推荐
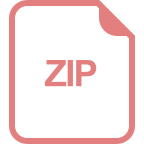
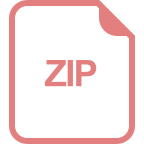
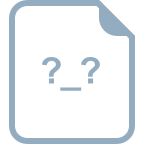
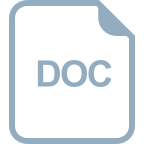
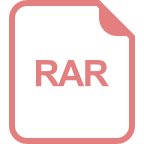
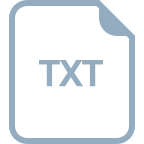
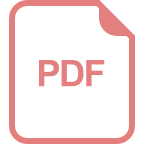
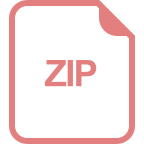
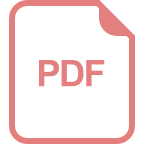
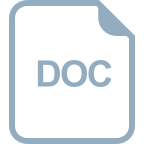
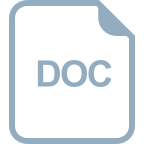
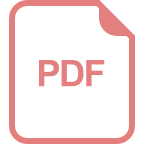
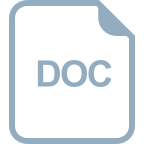
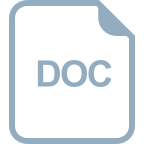


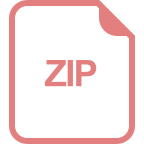