golang 调用harbor api删除镜像
时间: 2023-08-17 08:03:03 浏览: 55
要使用Golang调用Harbor API删除镜像,可以按照以下步骤进行操作:
1. 导入相关的Golang软件包:
```go
import (
"bytes"
"net/http"
)
```
2. 构建HTTP请求:
```go
func createRequest(method, url string, payload []byte) (*http.Request, error) {
req, err := http.NewRequest(method, url, bytes.NewBuffer(payload))
if err != nil {
return nil, err
}
req.Header.Set("Content-Type", "application/json")
return req, nil
}
```
3. 发送HTTP请求并获取响应:
```go
func sendRequest(req *http.Request) (*http.Response, error) {
client := http.DefaultClient
resp, err := client.Do(req)
if err != nil {
return nil, err
}
return resp, nil
}
```
4. 构建删除镜像的URL:
```go
func buildDeleteURL(baseURL, projectName, repoName, tag string) string {
return fmt.Sprintf("%s/api/repositories/%s/%s/tags/%s", baseURL, projectName, repoName, tag)
}
```
5. 执行删除镜像的操作:
```go
func deleteImage(baseURL, projectName, repoName, tag, username, password string) error {
url := buildDeleteURL(baseURL, projectName, repoName, tag)
req, err := createRequest("DELETE", url, nil)
if err != nil {
return err
}
req.SetBasicAuth(username, password)
resp, err := sendRequest(req)
if err != nil {
return err
}
defer resp.Body.Close()
if resp.StatusCode != http.StatusOK {
return fmt.Errorf("Failed to delete image. Status code: %d", resp.StatusCode)
}
return nil
}
```
其中,baseURL是Harbor的URL,projectName是项目名称,repoName是镜像仓库名称,tag是镜像标签,username和password是Harbor API的登录凭证。
通过以上步骤,就可以使用Golang调用Harbor API删除镜像了。
相关推荐
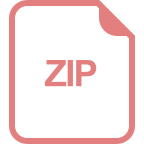
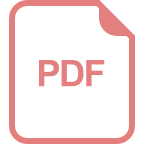
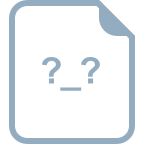














