判断由大小写字母和空格组成的字符串在忽略大小写和空格的情况下是否相等
时间: 2024-10-25 19:15:26 浏览: 26
要判断由大小写字母和空格组成的字符串在忽略大小写和空格的情况下是否相等,可以按照以下步骤进行:
1. **移除空格**:首先,从两个字符串中移除所有的空格。
2. **转换为小写或大写**:将处理后的字符串全部转换为小写或大写,以忽略大小写的差异。
3. **比较字符串**:最后,比较处理后的两个字符串是否相等。
下面是一个用C++实现的示例代码:
```cpp
#include <iostream>
#include <algorithm>
#include <cctype>
// 辅助函数:移除字符串中的空格并转换为小写
std::string preprocess(const std::string& str) {
std::string result;
for (char ch : str) {
if (!isspace(ch)) {
result += tolower(ch);
}
}
return result;
}
// 主函数:判断两个字符串在忽略大小写和空格的情况下是否相等
bool areEqualIgnoringCaseAndSpaces(const std::string& str1, const std::string& str2) {
return preprocess(str1) == preprocess(str2);
}
int main() {
std::string str1 = "Hello World";
std::string str2 = "h e llo w orld";
if (areEqualIgnoringCaseAndSpaces(str1, str2)) {
std::cout << "The strings are equal." << std::endl;
} else {
std::cout << "The strings are not equal." << std::endl;
}
return 0;
}
```
### 解释:
1. **preprocess函数**:这个函数遍历输入字符串,移除所有空格并将字符转换为小写。
2. **areEqualIgnoringCaseAndSpaces函数**:这个函数调用`preprocess`对两个字符串进行处理,然后比较处理后的结果。
3. **main函数**:测试了两个字符串,输出它们是否在忽略大小写和空格的情况下相等。
通过这种方式,你可以有效地判断两个字符串在忽略大小写和空格的情况下是否相等。
阅读全文
相关推荐
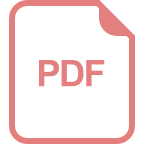
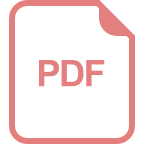















