java有界优先队列
时间: 2023-11-10 07:48:30 浏览: 224
Java中有界优先队列可以通过自定义类BoundedPriorityQueue来实现。该类继承自PriorityQueue类,并在构造函数中接收一个容量参数和一个比较器对象,用于设置队列的容量和自定义优先级规则。
举个例子,假设我们定义了一个Animal类实现了Comparable接口,并在compareTo方法中根据age字段从小到大排序。然后我们创建一个优先队列PriorityQueue来存储Animal对象。当我们添加Animal对象时,队列会根据compareTo方法的返回值进行排序,从而实现按照age从小到大的优先级。
如果我们想要创建一个有界的优先队列,我们可以使用BoundedPriorityQueue类并传递一个容量参数作为构造函数的参数。这样,当队列中的元素数量达到容量上限时,再次添加元素会触发队列中最小的元素被移除,从而保持队列的大小不超过给定的容量。
在上述例子中,我们可以使用BoundedPriorityQueue类来创建一个容量为5的有界优先队列,传递一个比较器对象来设置自定义的优先级规则。
相关问题
java ThreadPoolExecutor 队列
`ThreadPoolExecutor`是Java并发包中用于管理固定大小线程池的组件,它允许你创建一个线程池,并定义了任务的提交、执行以及资源的管理规则。队列(queue)在`ThreadPoolExecutor`中扮演着至关重要的角色,因为它存储待执行的任务,直到线程可用。
`ThreadPoolExecutor`支持多种队列策略,主要的有以下几种:
1. **ArrayBlockingQueue**:基于数组的有界阻塞队列,当队列满时,新任务会阻塞,直到其他任务完成并腾出空间。
2. **LinkedBlockingQueue**:基于链表的无界阻塞队列,任务的添加和移除操作都是O(1)时间复杂度,适合处理不确定的任务数量。
3. **PriorityBlockingQueue**:优先级队列,任务按照优先级排序,高优先级的任务优先执行。
4. **SynchronousQueue**:同步队列,没有容量,每次插入都会阻塞,直到有线程从队列移除,保证了任务的同步执行。
5. **DelayedWorkQueue**:用于延迟执行的任务队列,结合`DelayQueue`和`ThreadPoolExecutor`,适合执行延迟的任务。
6. 用户自定义`BlockingQueue`:如果你有自己的需求,还可以实现`BlockingQueue`接口并提供一个实例给`ThreadPoolExecutor`。
选择哪种队列取决于你的具体应用场景,比如任务是否需要按顺序执行、是否需要限制任务数量、是否关注任务优先级等。在配置`ThreadPoolExecutor`时,你需要指定`workQueue`参数来指定队列实现。
阅读全文
相关推荐
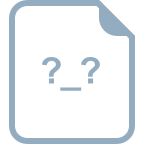
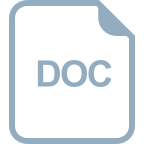
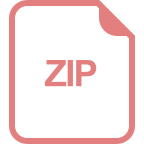
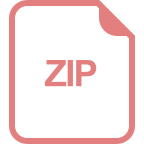
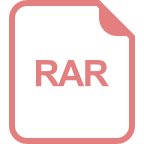
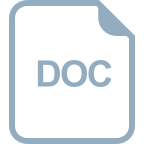
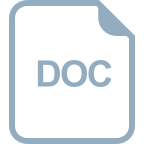
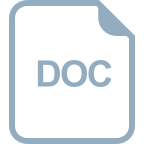
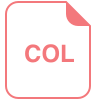
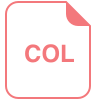
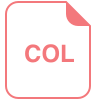
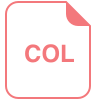
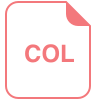
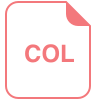
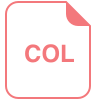