创建二叉树,遍历二叉树、求二叉树叶子节点数、求二叉树深度
时间: 2024-12-25 11:29:39 浏览: 16
创建二叉树通常需要定义一个节点类,包含两个子节点引用和一个值。例如,在Python中,可以这样定义:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
```
遍历二叉树常见的有三种方式:
1. **前序遍历** (根-左-右): 访问根节点,然后递归地访问左子树,最后访问右子树。
2. **中序遍历** (左-根-右): 先递归地访问左子树,然后访问根节点,最后访问右子树。
3. **后序遍历** (左-右-根): 先递归地访问左子树和右子树,最后访问根节点。
计算叶子节点数可以通过遍历过程中计数来完成,如果当前节点的左右子节点都为空,则它是叶子节点。
```python
def count_leaves(node):
if node is None:
return 0
elif node.left is None and node.right is None:
return 1
else:
return count_leaves(node.left) + count_leaves(node.right)
```
求二叉树的深度也通过递归,返回从根到最深叶子节点的最大距离:
```python
def tree_depth(root):
if root is None:
return 0
else:
left_depth = tree_depth(root.left)
right_depth = tree_depth(root.right)
return max(left_depth, right_depth) + 1
```
阅读全文
相关推荐







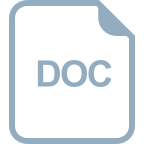






