下面有结构node的定义,指针head中存放着node节点组成 的单链表的首节点地址,函数odd(head)获取head指向链表 中的序号(注意不是node结构的data字段,且序号从0开始编号) 为奇数的节点,并将这些节点按原有序组成新链表返回。 要求新链表中的节点采用动态内存分配的方式构建, 从而不破坏原链表。如:head指向的链表为:1->2->3->4->5->6, 则odd(head)得到的链表为:2->4->6。请完成函数odd的编写。
时间: 2024-04-14 12:27:52 浏览: 22
以下是函数odd的实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* odd(Node* head) {
// 创建新链表的头指针和尾指针
Node* newHead = NULL;
Node* newTail = NULL;
Node* current = head;
int index = 0;
while (current != NULL) {
if (index % 2 != 0) { // 如果序号为奇数
// 创建新节点
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = current->data;
newNode->next = NULL;
if (newHead == NULL) { // 如果新链表为空
newHead = newNode;
newTail = newNode;
} else {
newTail->next = newNode;
newTail = newNode;
}
}
current = current->next;
index++;
}
return newHead;
}
```
使用示例:
```c
int main() {
// 创建原链表
Node* head = (Node*)malloc(sizeof(Node));
head->data = 1;
Node* node1 = (Node*)malloc(sizeof(Node));
node1->data = 2;
head->next = node1;
Node* node2 = (Node*)malloc(sizeof(Node));
node2->data = 3;
node1->next = node2;
Node* node3 = (Node*)malloc(sizeof(Node));
node3->data = 4;
node2->next = node3;
Node* node4 = (Node*)malloc(sizeof(Node));
node4->data = 5;
node3->next = node4;
Node* node5 = (Node*)malloc(sizeof(Node));
node5->data = 6;
node4->next = node5;
node5->next = NULL;
// 调用odd函数获取新链表
Node* newHead = odd(head);
// 打印新链表
Node* current = newHead;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
// 释放内存
current = newHead;
while (current != NULL) {
Node* temp = current;
current = current->next;
free(temp);
}
return 0;
}
```
输出结果为:
```
2 4 6
```
相关推荐
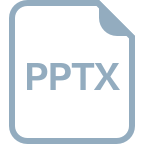
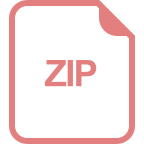









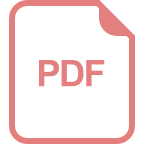
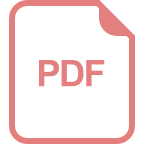
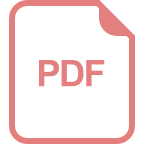
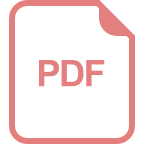
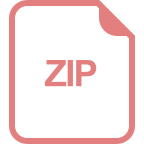