用c语言将一个链表分解成按顺序奇偶两个链表的具体代码
时间: 2023-07-12 19:31:55 浏览: 71
下面是一个用C语言将一个链表分解成按顺序奇偶两个链表的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* oddEvenList(struct ListNode* head) {
if (!head) return NULL;
struct ListNode *odd = head, *even = head->next, *even_head = even;
while (even && even->next) {
odd = odd->next = even->next;
even = even->next = odd->next;
}
odd->next = even_head;
return head;
}
// 创建链表
struct ListNode* createList(int* nums, int numsSize) {
struct ListNode *head = NULL, *tail = NULL;
for (int i = 0; i < numsSize; i++) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = nums[i];
node->next = NULL;
if (!head) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
// 打印链表
void printList(struct ListNode* head) {
while (head) {
printf("%d ", head->val);
head = head->next;
}
printf("\n");
}
int main() {
int nums[] = {1, 2, 3, 4, 5};
int numsSize = sizeof(nums) / sizeof(nums[0]);
struct ListNode* head = createList(nums, numsSize);
printf("原链表:");
printList(head);
head = oddEvenList(head);
printf("奇偶链表:");
printList(head);
return 0;
}
```
该代码中,我们首先定义了一个链表节点结构体 `struct ListNode`,然后实现了一个函数 `oddEvenList`,用于将一个链表分解成按顺序奇偶两个链表。具体实现上,我们使用了两个指针 odd 和 even,分别表示奇数和偶数节点的链表头。然后我们遍历链表,将奇数节点和偶数节点分别连接到 odd 和 even 链表上,最后将 odd 链表的尾部连接到 even 链表的头部即可。
我们还实现了一个 `createList` 函数用于创建链表,以及一个 `printList` 函数用于打印链表。最后在 `main` 函数中,我们创建了一个示例链表,然后调用 `oddEvenList` 函数将其分解为奇偶链表,并打印输出结果。
相关推荐
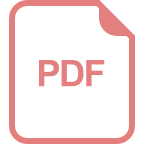














