import os import csv from bs4 import BeautifulSoup # 文件夹路径 folder_path = 'C:\Users\test\Desktop\DIDItest' # CSV文件路径 csv_file = r'C:\Users\test\Desktop\output.csv' # 创建CSV文件并写入表头 with open(csv_file, 'w', newline='', encoding='utf-8') as file: writer = csv.writer(file) writer.writerow(['ID', '时间', '发送号码', '接收号码', '发送内容']) # 遍历文件夹内的所有文件 for file_name in os.listdir(folder_path): file_path = os.path.join(folder_path, file_name) # 判断文件是否为HTML文件 if file_name.endswith('.html'): # 打开HTML文件并解析源代码 with open(file_path, 'r', encoding='utf-8') as file: soup = BeautifulSoup(file, 'html.parser') # 提取ID、时间、发送号码、接收号码和发送内容 talk_id = soup.find('span', class_='hint-success').text.strip() time = soup.find('body').contents[0].strip() send_number = soup.find_all('span', class_='hint-success')[0].text.strip() receive_number = soup.find_all('span', class_='hint-success')[1].text.strip() content = soup.find('p').contents[0].strip() # 判断发送内容是否为音频 if '音频' in content: audio_link = soup.find('a')['href'] content = f'音频文件位置:{audio_link}' # 将提取的信息写入CSV文件 with open(csv_file, 'a', newline='', encoding='utf-8') as file: writer = csv.writer(file) writer.writerow([talk_id, time, send_number, receive_number, content]) print("数据已成功写入CSV文件。")
时间: 2024-01-29 10:02:54 浏览: 28
代码看起来没有问题,但是有几个地方需要注意:
1. 在Windows中,文件路径使用反斜杠`\`作为路径分隔符。请确保将`folder_path`和`csv_file`中的路径分隔符改为`\\`,或者使用原始字符串(在字符串前面加上`r`)。
2. 请确保文件夹路径和CSV文件路径是正确的,并且有读取和写入的权限。
如果你仍然遇到乱码问题,请提供更多关于乱码的详细信息,例如乱码的具体样式或错误信息,以便我能更好地帮助你解决问题。
相关问题
import os import re import csv from bs4 import BeautifulSoup folder_path = 'C:/Users/test/Desktop/DIDItest' html_files = [] # 遍历文件夹及其子文件夹下所有HTML文件 for root, dirs, files in os.walk(folder_path): for file in files: if file.endswith('.html'): html_files.append(os.path.join(root, file))打开并提取所有html文件源代码
您好!要打开并提取所有HTML文件的源代码,可以按照以下步骤进行操作:
1. 导入所需的库:
```python
import os
from bs4 import BeautifulSoup
```
2. 指定文件夹路径和一个空列表来存储HTML文件的路径:
```python
folder_path = 'C:/Users/test/Desktop/DIDItest'
html_files = []
```
3. 使用`os.walk()`函数遍历文件夹及其子文件夹下的所有文件,并筛选出以`.html`结尾的文件:
```python
for root, dirs, files in os.walk(folder_path):
for file in files:
if file.endswith('.html'):
html_files.append(os.path.join(root, file))
```
4. 循环遍历所有HTML文件,并使用BeautifulSoup库打开并提取源代码:
```python
for file_path in html_files:
with open(file_path, 'r', encoding='utf-8') as file:
html_source_code = file.read()
soup = BeautifulSoup(html_source_code, 'html.parser')
# 在这里可以对源代码进行进一步的处理或提取需要的信息
```
请注意,这里使用了BeautifulSoup库来解析HTML文件,您需要确保已经安装了该库,可以使用`pip install beautifulsoup4`来安装。
希望能对您有所帮助!如有任何疑问,请随时提问。
import os import csv from bs4 import BeautifulSoup # 设置文件夹路径 folder_path = 'C:/Users/test/Desktop/DIDItest' # 遍历文件夹中的所有文件和子文件夹 for root, dirs, files in os.walk(folder_path): for file_name in files: if file_name.endswith('.html'): file_path = os.path.join(root, file_name) # 打开HTML文件并读取源代码 with open(file_path, 'r', encoding='utf-8') as file: html_content = file.read() # 在这里可以对源代码进行进一步的操作和提取 soup = BeautifulSoup(html_content, 'html.parser') messages = soup.find_all('p') for message in messages: talk_id_tag = message.find_previous('a') if talk_id_tag: talk_id = talk_id_tag.text.strip()[1:] time = message.contents[0].strip().split(',')[0][1:] send_phone = message.find('span', class_='hint-success').text.strip() receive_phone = message.find_all('span', class_='hint-success')[1].text.strip() content_tag = message.find('a') if content_tag: content = content_tag['href'] content_type = '音频' else: content = message.text.strip() content_type = '文本' print("talkid:", talk_id) print("时间:", time) print("发送号码:", send_phone) print("接收号码:", receive_phone) print("发送内容:", content) print()
这段代码有一个缩进问题,最后的`print`语句没有在正确的位置,应该在`for`循环内,以便在每次迭代时打印出提取的信息。请将最后的`print`语句缩进到正确的位置。以下是修改后的代码:
```python
import os
import csv
from bs4 import BeautifulSoup
# 设置文件夹路径
folder_path = 'C:/Users/test/Desktop/DIDItest'
# 遍历文件夹中的所有文件和子文件夹
for root, dirs, files in os.walk(folder_path):
for file_name in files:
if file_name.endswith('.html'):
file_path = os.path.join(root, file_name)
# 打开HTML文件并读取源代码
with open(file_path, 'r', encoding='utf-8') as file:
html_content = file.read()
# 在这里可以对源代码进行进一步的操作和提取
soup = BeautifulSoup(html_content, 'html.parser')
messages = soup.find_all('p')
for message in messages:
talk_id_tag = message.find_previous('a')
if talk_id_tag:
talk_id = talk_id_tag.text.strip()[1:]
time = message.contents[0].strip().split(',')[0][1:]
send_phone = message.find('span', class_='hint-success').text.strip()
receive_phone = message.find_all('span', class_='hint-success')[1].text.strip()
content_tag = message.find('a')
if content_tag:
content = content_tag['href']
content_type = '音频'
else:
content = message.text.strip()
content_type = '文本'
print("talkid:", talk_id)
print("时间:", time)
print("发送号码:", send_phone)
print("接收号码:", receive_phone)
print("发送内容:", content)
print()
```
请注意,这段代码还没有将提取到的信息写入CSV文件,你可以根据需要进一步完善代码来实现写入CSV的功能。
相关推荐
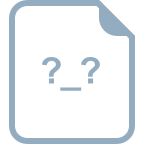
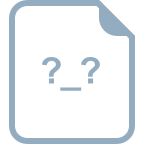






import os from bs4 import BeautifulSoup import re # 指定文件夹路径 folder_path = "C:/Users/test/Desktop/DIDItest" # 正则表达式模式 pattern = r'<body>(.*?)<\/body>' # 遍历文件夹中的所有文件 for root, dirs, files in os.walk(folder_path): for file in files: # 读取html文件 file_path = os.path.join(root, file) with open(file_path, "r", encoding="utf-8") as f: html_code = f.read() # 使用正则表达式匹配<body>标签内的数据 body_data = re.findall(pattern, html_code, re.DOTALL) # 剔除和() body_data = body_data[0].replace("", "").replace("()", "") # 使用正则表达式提取talk_id、时间、发送者ID和接收者ID matches = re.findall(r'\[talkid:(\d+)\](\d+年\d+月\d+日 \d+:\d+:\d+).*?<span.*?>(\d+)<.*?>(.*?)<', body_data) # 提取唯一ID,时间,发送号码和私聊群聊关键词 matches1 = re.findall(r'<span.*?hint-success.*?>(\d+)<.*?>', body_data) # match = re.search('(中发言|发送)\s(.*?)\s', body_data) # if match: # content = match.group(2) matches2 = re.findall('(中发言|发送)\s(.*?)\s', body_data) for match in matches2: content = match[1] soup = BeautifulSoup(content, 'html.parser') if soup.find('= 2: receive_id = matches1[3] # 处理匹配结果 for match in matches: talk_id = match[0] time = match[1] send_id = match[2] talk_type = match[3] # 进行时间格式转换,将time转换为"0000-00-00"格式 time = time.replace('年', '-').replace('月', '-').replace('日', '') talk_type = talk_type.replace('向', '私聊').replace('在群', '群聊') # 打印结果 print("Talk ID:", talk_id) print("Time:", time) print("Sender ID:", send_id) print("Receive_id:", receive_id) print("Talk_type:", talk_type) print("Content:",content) print("---")导入至csv





利用python爬虫,提取C:/Users/test/Desktop/DIDItest文件夹下每个文件夹内的html文件源代码,并提取源代码中的ID、时间、发送号码、接收号码、发送内容,如果发送内容为音频则提取音频所在位置,反之则保留发送内容,并将爬取的内容写入csv中 网页内源代码如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031361]2014年4月20日 03:55:45 , 434343 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031362]2014年4月20日 04:45:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031363]2014年4月20日 04:55:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () [talkid:138031364]2014年4月20日 05:55:45 , 434343 向 3234221 发送 我们已经是好友了,开始聊天吧! () [talkid:138031365]2014年4月20日 06:55:45 , 434343 向 1359075 发送 我们已经是好友了,开始聊天吧! () </body> </html>
利用python爬虫,提取C:/Users/test/Desktop/DIDItest文件夹下多个文件内的html文件源代码,并提取源代码中的ID、时间、发送号码、接收号码、发送内容,如果发送内容为音频则提取音频所在位置,反之则保留发送内容,并将爬取的内容写入csv中 网页内源代码如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031361]2014年4月20日 03:55:45 , 434343 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031362]2014年4月20日 04:45:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031363]2014年4月20日 04:55:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () [talkid:138031364]2014年4月20日 05:55:45 , 434343 向 3234221 发送 我们已经是好友了,开始聊天吧! () [talkid:138031365]2014年4月20日 06:55:45 , 434343 向 1359075 发送 我们已经是好友了,开始聊天吧! () </body> </html>
网页内源代码模板如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031370]2014年4月20日 03:55:45 , 111222 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031371]2014年4月20日 04:45:45 , 111222 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031372]2014年4月20日 04:55:45 , 111222 向 123456 发送 图片 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () </body> </html> 利用python爬虫,打开C:/Users/test/Desktop/DIDItest文件夹下多个文件夹内的html文件源代码,并将源代码转换为字符串,爬取源代码字符串中的ID、时间、发送号码、接收号码、信息类型、发送内容,如果发送内容不为文本,则提取文件所在链接地址,并将爬取的内容写入csv中,talkid提取[]中talkid:后的数字、时间精确至年月日时分秒、发送号码提取第一个 data-hint"">之间的数字,接收号码提取第二个data-hint"">,信息类型就提取 发送与:之间的文字,如果没有:则定义为文字