蚁群算法代码加解释、
时间: 2024-11-08 15:13:05 浏览: 37
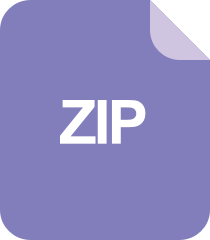
蚁群算法C程序代码.zip
下面是一个简单的Python蚁群算法示例,使用了`networkx`库来构建网络图。这个例子演示了如何在一个二维网格上寻找两点之间的最短路径。
```python
import numpy as np
import networkx as nx
# 初始化蚂蚁群系统
def init_ants(grid_size, num_ants):
ants = [(i, j) for i in range(grid_size) for j in range(grid_size)]
start, end = (0, 0), (grid_size - 1, grid_size - 1)
return ants, start, end
# 计算邻居节点
def get_neighbors(node, grid_size):
x, y = node
neighbors = [(x + dx, y + dy) for dx, dy in [(-1, 0), (1, 0), (0, -1), (0, 1)] if 0 <= x + dx < grid_size and 0 <= y + dy < grid_size]
return neighbors
# 判断是否到达终点
def is_goal(node, end):
return node == end
# 更新信息素
def update_pheromone(pheromone_matrix, path, alpha=1, beta=1):
for node in path[1:]:
pheromone_matrix[node] += 1 / len(path)
# 寻找路径
def ant_colony_search(ants, start, end, pheromone_matrix, max_iterations=100):
best_path = None
for _ in range(max_iterations):
# 随机选择一个蚂蚁和下一个移动节点
ant, current_node = np.random.choice(ants), start
path = [current_node]
while not is_goal(current_node, end):
unvisited_neighbors = get_neighbors(current_node, len(pheromone_matrix))
probabilities = [(pheromone[i] ** alpha) * (1 / np.sum([pheromone[j] for j in unvisited_neighbors])) for i in unvisited_neighbors]
next_node = np.random.choice(unvisited_neighbors, p=probabilities)
path.append(next_node)
current_node = next_node
# 更新信息素
update_pheromone(pheromone_matrix, path)
if not best_path or len(path) < len(best_path):
best_path = path
return best_path
# 示例
grid_size = 8
ants, start, end = init_ants(grid_size, 50)
pheromone_matrix = np.zeros((grid_size, grid_size))
best_path = ant_colony_search(ants, start, end, pheromone_matrix)
print("Best found path:", best_path, "from", start, "to", end)
```
在这个例子中,我们首先初始化了一个二维网格,并生成了一些蚂蚁。每只蚂蚁会在网格上按照信息素浓度的概率选择下一个节点,直到找到目标点。信息素矩阵表示了每个节点的吸引力,随着路径被多次发现而逐渐增强。
阅读全文
相关推荐
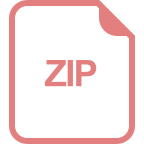
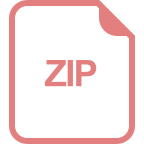
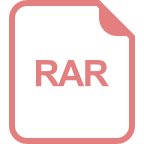
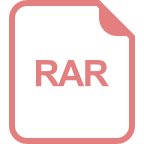
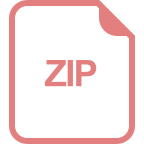
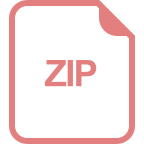
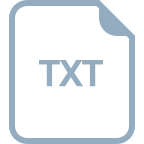
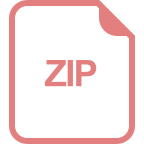
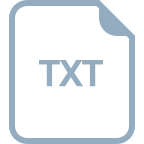
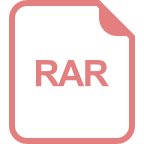
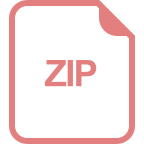
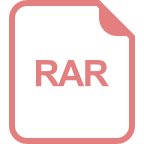
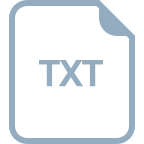
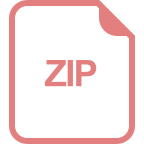
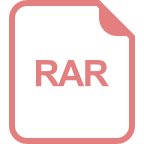
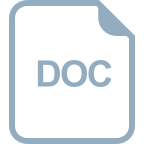
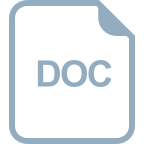
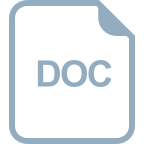