java爱心烟花代码
时间: 2023-12-03 21:41:19 浏览: 161
以下是Java实现爱心烟花的代码:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Fireworks extends JPanel implements Runnable {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
private static final int FIREWORKS_NUM = 10;
private static final int FIREWORKS_PARTICLE_NUM = 100;
private static final int FIREWORKS_PARTICLE_SIZE = 5;
private static final int FIREWORKS_PARTICLE_SPEED = 10;
private static final int FIREWORKS_EXPLODE_SPEED = 5;
private static final int FIREWORKS_EXPLODE_SIZE = 20;
private static final int FIREWORKS_EXPLODE_PARTICLE_SIZE = 3;
private static final int FIREWORKS_EXPLODE_PARTICLE_SPEED = 5; private static final int FIREWORKS_EXPLODE_PARTICLE_NUM = 100;
private static final int FIREWORKS_EXPLODE_PARTICLE_LIFE = 100;
private static final int FIREWORKS_EXPLODE_PARTICLE_FADE = 5;
private static final int FIREWORKS_DELAY = 50;
private List<Firework> fireworks = new ArrayList<Firework>();
public Fireworks() {
setPreferredSize(new java.awt.Dimension(WIDTH, HEIGHT));
setBackground(Color.BLACK);
for (int i = 0; i < FIREWORKS_NUM; i++) {
fireworks.add(new Firework());
}
}
@Override
public void run() {
while (true) {
try {
Thread.sleep(FIREWORKS_DELAY);
} catch (InterruptedException e) {
e.printStackTrace();
}
repaint();
}
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
for (Firework firework : fireworks) {
firework.draw(g);
}
}
private class Firework {
private Point position;
private Point velocity;
private List<Particle> particles = new ArrayList<Particle>(); private boolean exploded = false;
public Firework() {
position = new Point((int) (Math.random() * WIDTH), HEIGHT);
velocity = new Point(0, -(int) (Math.random() * FIREWORKS_PARTICLE_SPEED));
}
public void draw(Graphics g) {
if (!exploded) {
g.setColor(Color.WHITE);
g.fillRect(position.x, position.y, FIREWORKS_PARTICLE_SIZE, FIREWORKS_PARTICLE_SIZE);
move();
if (velocity.y >= 0) {
explode();
}
} else {
for (Particle particle : particles) {
particle.draw(g);
}
}
}
private void move() {
position.x += velocity.x;
position.y += velocity.y;
velocity.y += 1;
}
private void explode() {
exploded = true;
for (int i = 0; i < FIREWORKS_EXPLODE_PARTICLE_NUM; i++) {
particles.add(new Particle(position));
}
}
private class Particle {
private Point position;
private Point velocity;
private int life;
public Particle(Point position) {
this.position = new Point(position);
velocity = new Point((int) (Math.random() * FIREWORKS_EXPLODE_PARTICLE_SPEED * 2 - FIREWORKS_EXPLODE_PARTICLE_SPEED),
(int) (Math.random() * FIREWORKS_EXPLODE_PARTICLE_SPEED * 2 - FIREWORKS_EXPLODE_PARTICLE_SPEED));
life = FIREWORKS_EXPLODE_PARTICLE_LIFE;
}
public void draw(Graphics g) {
g.setColor(new Color((int) (Math.random() * 255), (int) (Math.random() * 255), (int) (Math.random() * 255), life));
g.fillRect(position.x, position.y, FIREWORKS_EXPLODE_PARTICLE_SIZE, FIREWORKS_EXPLODE_PARTICLE_SIZE);
move();
}
private void move() {
position.x += velocity.x;
position.y += velocity.y;
life -= FIREWORKS_EXPLODE_PARTICLE_FADE;
}
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Fireworks");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Fireworks fireworks = new Fireworks();
frame.getContentPane().add(fireworks);
frame.pack();
frame.setVisible(true);
new Thread(fireworks).start();
}
}
```
阅读全文
相关推荐




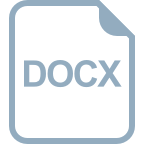
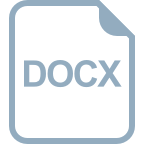
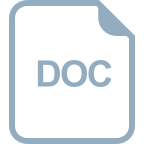


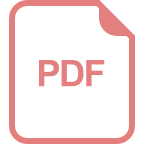
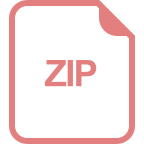
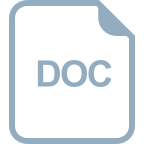
