python编写程序,首先对图像添加椒盐噪声或高斯噪声,然后对加噪图像进行均值滤波、中值滤波和高斯滤波
时间: 2024-09-26 18:12:50 浏览: 47
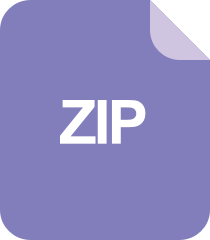
图像滤波_图像去噪椒盐噪声高斯噪声_

在Python中,处理图像并添加噪声以及进行滤波通常涉及使用像OpenCV(Open Source Computer Vision Library)这样的库。以下是基本步骤:
1. **安装必要的库**:
首先,你需要安装`opencv-python`和其他可能需要的数据科学库,比如`numpy`。可以使用pip命令安装:
```bash
pip install opencv-python numpy
```
2. **导入所需的模块**:
导入OpenCV和其他所需的模块:
```python
import cv2
import numpy as np
```
3. **添加噪声**:
- **椒盐噪声(Salt and Pepper Noise)**:
```python
salt_vs_pepper = 0.05 # 设置噪声比例
noisy_image = cv2.imread('image.jpg', 0) + np.random.randint(-255*salt_vs_pepper, 255*salt_vs_pepper, size=noisy_image.shape, dtype=np.uint8)
```
- **高斯噪声(Gaussian Noise)**:
```python
gaussian_noise = np.random.normal(0, 40, noisy_image.shape) # mean=0, std deviation (sigma), shape of image
noisy_image += gaussian_noise
```
4. **滤波处理**:
- **均值滤波**:
```python
blur = cv2.blur(noisy_image, (5, 5)) # kernel size is a tuple
```
- **中值滤波**:
```python
median_blur = cv2.medianBlur(noisy_image, 5)
```
- **高斯滤波**:
```python
gaussian_blur = cv2.GaussianBlur(noisy_image, (5, 5), 0)
```
5. **显示原图与处理后的图像**:
```python
cv2.imshow('Original Image', noisy_image)
cv2.imshow('Filtered Image (Mean)', blur)
cv2.imshow('Filtered Image (Median)', median_blur)
cv2.imshow('Filtered Image (Gaussian)', gaussian_blur)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
记得替换`'image.jpg'`为你要处理的图片路径。
阅读全文
相关推荐
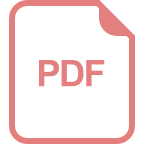






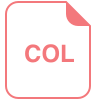







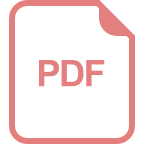
