模拟简单的商品管理系统 题目描述:定义 Product 类,包含 id、name、price 等属性,创建一个商品列表,并实现查询 和添加商品的功能。
时间: 2024-10-11 11:15:07 浏览: 54
模拟一个简单的商品管理系统,我们可以首先创建一个 `Product` 类,它包含基本的商品信息属性,如ID、名称和价格。然后,我们可以使用一个数据结构,比如 List 或 HashMap,来管理商品列表。
```java
import java.util.ArrayList;
import java.util.HashMap;
class Product {
private int id;
private String name;
private double price;
public Product(int id, String name, double price) {
this.id = id;
this.name = name;
this.price = price;
}
// getter 和 setter 方法
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
public class SimpleProductManager {
private ArrayList<Product> productList; // 商品列表,可以使用 HashMap 替换为键值对形式
public SimpleProductManager() {
productList = new ArrayList<>();
}
// 添加商品方法
public void addProduct(Product product) {
productList.add(product);
}
// 查询商品方法,这里简化为按 ID 查找
public Product getProductById(int productId) {
for (Product p : productList) {
if (p.getId() == productId) {
return p;
}
}
return null; // 如果找不到,则返回 null
}
// 示例用法
public static void main(String[] args) {
SimpleProductManager manager = new SimpleProductManager();
Product apple = new Product(1, "苹果", 5.99);
manager.addProduct(apple);
Product banana = new Product(2, "香蕉", 2.99);
manager.addProduct(banana);
Product foundProduct = manager.getProductById(1); // 获取 ID 为 1 的商品
if (foundProduct != null) {
System.out.println("找到了产品:" + foundProduct.getName());
} else {
System.out.println("未找到该产品");
}
}
}
```
在这个例子中,`SimpleProductManager` 类有一个商品列表,通过 `addProduct` 方法添加商品,`getProductById` 方法用于根据 ID 查询商品。如果商品存在,就会返回对应的 `Product` 对象;否则,返回 `null`。
阅读全文
相关推荐
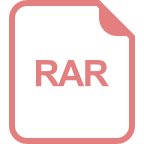
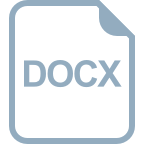
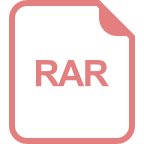












