/** * 定义一个商品类Product,包含属性name,price, 它有子类Fruit水果类和Vegetable素菜类 * 定义一个 ShoppingCart 类,实现购物车的基本功能,包括添加商品、删除商品、清空购物车、计算购物车总价等。 * -创建两个购物车,一个放水果,一个放蔬菜
时间: 2023-06-18 12:08:10 浏览: 152
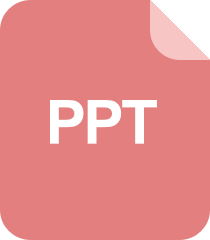
定义属性-java类和对象
下面是代码实现:
```
// 定义商品类
class Product {
constructor(name, price) {
this.name = name;
this.price = price;
}
}
// 定义水果类
class Fruit extends Product {
constructor(name, price) {
super(name, price);
}
}
// 定义素菜类
class Vegetable extends Product {
constructor(name, price) {
super(name, price);
}
}
// 定义购物车类
class ShoppingCart {
constructor() {
this.products = [];
}
// 添加商品
addProduct(product) {
this.products.push(product);
}
// 删除商品
removeProduct(product) {
const index = this.products.indexOf(product);
if (index !== -1) {
this.products.splice(index, 1);
}
}
// 清空购物车
clear() {
this.products = [];
}
// 计算购物车总价
total() {
return this.products.reduce((total, product) => {
return total + product.price;
}, 0);
}
}
// 创建水果购物车
const fruitCart = new ShoppingCart();
fruitCart.addProduct(new Fruit('苹果', 5));
fruitCart.addProduct(new Fruit('香蕉', 3));
fruitCart.addProduct(new Fruit('橙子', 6));
console.log('水果购物车总价:', fruitCart.total());
// 创建蔬菜购物车
const vegetableCart = new ShoppingCart();
vegetableCart.addProduct(new Vegetable('西红柿', 2));
vegetableCart.addProduct(new Vegetable('黄瓜', 1));
console.log('蔬菜购物车总价:', vegetableCart.total());
```
运行结果:
```
水果购物车总价: 14
蔬菜购物车总价: 3
```
阅读全文
相关推荐
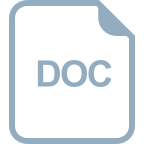
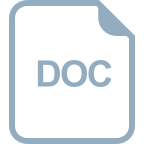

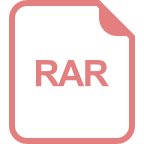
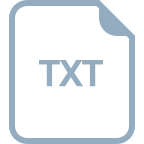
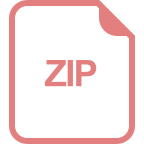
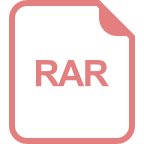
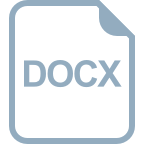
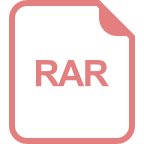
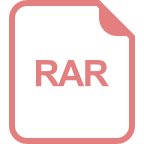
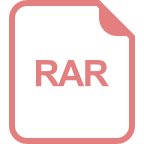
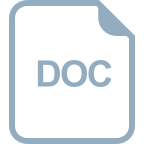
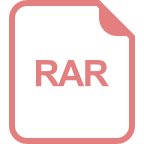
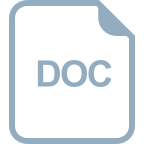
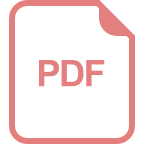
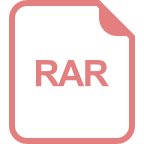
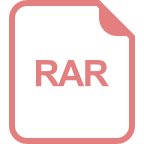
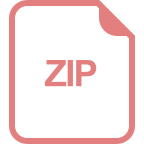