https://e.weather.com.cn/mweather/101230605.shtml,爬取当前天气信息(包括地址温度等)存储到csv文件中
时间: 2025-01-06 19:47:48 浏览: 4
要爬取天气信息并将其存储到CSV文件中,可以使用Python的`requests`库来发送HTTP请求,`BeautifulSoup`库来解析HTML内容,`csv`库来写入CSV文件。以下是一个示例代码:
```python
import requests
from bs4 import BeautifulSoup
import csv
# 目标URL
url = "https://e.weather.com.cn/mweather/101230605.shtml"
# 发送HTTP请求
response = requests.get(url)
response.encoding = 'utf-8' # 设置编码格式
# 解析HTML内容
soup = BeautifulSoup(response.text, 'html.parser')
# 提取天气信息
address = soup.find('div', class_='t').get_text(strip=True)
temperature = soup.find('div', class_='tem').get_text(strip=True)
weather = soup.find('div', class_='wea').get_text(strip=True)
# 打印提取的信息
print(f"地址: {address}")
print(f"温度: {temperature}")
print(f"天气: {weather}")
# 将信息写入CSV文件
with open('weather.csv', mode='w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['地址', '温度', '天气'])
writer.writerow([address, temperature, weather])
```
### 解释
1. **发送HTTP请求**:使用`requests.get`方法发送HTTP请求到目标URL。
2. **解析HTML内容**:使用`BeautifulSoup`解析返回的HTML内容。
3. **提取天气信息**:通过查找特定的HTML标签和类名提取地址、温度和天气信息。
4. **写入CSV文件**:使用`csv`库将提取的信息写入CSV文件。
### 注意事项
- **编码问题**:确保正确设置响应的编码格式,否则可能会出现乱码。
- **HTML结构变化**:网站的HTML结构可能会发生变化,导致代码无法正确提取信息。需要根据实际情况调整代码。
- **反爬虫机制**:一些网站可能有反爬虫机制,可能会阻止频繁的请求。可以使用`time.sleep`方法在请求之间添加延迟。
阅读全文
相关推荐
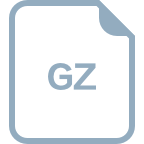



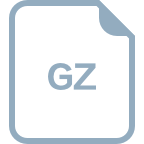
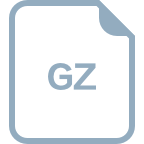





