c语言输入一行字符,计算该行字符中包含多少单词,单词之间用空格分开
时间: 2024-10-10 08:04:14 浏览: 37
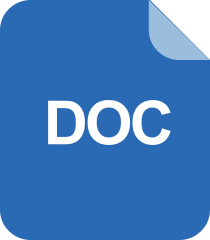
C语言图形编程.doc
在C语言中,你可以通过读取用户输入的一行字符串,并遍历这个字符串来计算其中包含的单词数。这里是一个简单的示例:
```c
#include <stdio.h>
#include <ctype.h> // 包含处理字符类型的函数
int count_words(char str[]) {
int word_count = 0;
bool in_word = false; // 标记是否正在处理单词
for (int i = 0; str[i] != '\0'; i++) { // 遍历字符串直到结束符
if (isspace(str[i])) { // 如果遇到空格
if (!in_word) continue; // 如果不是在单词内部,跳过
else word_count++; // 否则增加单词计数,表示找到了一个单词
in_word = false; // 结束当前单词
} else {
in_word = true; // 如果遇到非空格字符,则开始新的单词
}
}
// 检查最后一个单词是否终止于空格之外
if (!in_word && str[strlen(str) - 1] != ' ') {
word_count++;
}
return word_count;
}
int main() {
char input[100];
printf("请输入一行字符: ");
fgets(input, sizeof(input), stdin); // 从标准输入获取一行字符,包括换行符
input[strcspn(input, "\n")] = '\0'; // 移除多余的换行符
int word_count = count_words(input);
printf("该行字符中有 %d 个单词.\n", word_count);
return 0;
}
```
在这个程序中,`count_words` 函数会检查每个字符,如果遇到空格就判断是否在单词内,如果不是就在计数器加一。主函数部分则用于接收用户的输入并调用计数函数。
阅读全文
相关推荐
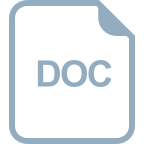
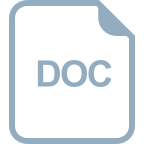















