1、输入一行字符,计算该行字符中包含多少个单词,单词之间用空格分隔开(c语言)
时间: 2024-10-19 07:09:55 浏览: 74
在C语言中,计算字符串中单词的数量通常需要将字符串按空格分割成单个单词,并统计元素个数。你可以通过以下几个步骤实现:
1. 定义一个函数,如`count_words(char *str)`,接收一个指向字符串的指针作为参数。
2. 创建一个变量`word_count`初始化为0,用于存储单词总数。
3. 使用`while`循环遍历字符串,直到遇到字符串结束(`'\0'`)。
a. 检查当前字符是否为空格(`isspace()`函数),如果是,则`word_count++`并继续检查下一个字符。
b. 如果不是空格,说明当前字符属于某个单词,继续扫描直到遇到空格。
4. 循环结束后,`word_count`就是单词的实际数目。
以下是简单的代码示例:
```c
#include <stdio.h>
#include <ctype.h>
int count_words(char *str) {
int word_count = 0;
while (*str != '\0') {
if (isspace(*str)) { // 空格判断
word_count++;
}
str++; // 移动到下一个字符
}
return word_count; // 返回单词计数
}
int main() {
char input[100];
printf("Enter a line of characters: ");
fgets(input, sizeof(input), stdin); // 获取用户输入
int word_count = count_words(input);
printf("The number of words in the string is: %d\n", word_count);
return 0;
}
```
阅读全文
相关推荐
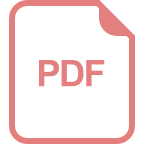
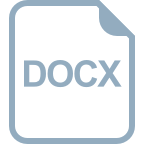















