LocalDateTime day +1
时间: 2023-11-28 09:44:44 浏览: 42
要将LocalDateTime加1天,可以使用plusDays()方法。以下是一个示例代码:
```java
LocalDateTime localDateTime = LocalDateTime.now();
LocalDateTime nextDay = localDateTime.plusDays(1);
System.out.println("Tomorrow is: " + nextDay);
```
这个代码将获取当前时间并将其存储在localDateTime变量中。然后,它使用plusDays()方法将localDateTime加1天,并将结果存储在nextDay变量中。最后,它将明天的日期打印出来。
相关问题
localdatetime
LocalDateTime 是 Java 8 中的一个日期时间类,表示本地日期和时间,不考虑时区的影响。它有许多方法可以获取和设置日期和时间的不同部分,例如年、月、日、小时、分钟和秒。它还可以执行日期和时间的算术运算,例如添加或减去一定的天数、小时或分钟。
以下是一个示例代码,演示如何创建和使用 LocalDateTime 类:
```
import java.time.LocalDateTime;
public class LocalDateTimeExample {
public static void main(String[] args) {
// 创建当前日期和时间的 LocalDateTime 对象
LocalDateTime now = LocalDateTime.now();
System.out.println("当前日期和时间:" + now);
// 从年、月、日、小时、分钟和秒创建 LocalDateTime 对象
LocalDateTime dateTime = LocalDateTime.of(2021, 7, 1, 10, 30, 0);
System.out.println("指定日期和时间:" + dateTime);
// 获取 LocalDateTime 对象的年、月、日、小时、分钟和秒
int year = now.getYear();
int month = now.getMonthValue();
int day = now.getDayOfMonth();
int hour = now.getHour();
int minute = now.getMinute();
int second = now.getSecond();
System.out.println("当前年份:" + year);
System.out.println("当前月份:" + month);
System.out.println("当前日期:" + day);
System.out.println("当前小时:" + hour);
System.out.println("当前分钟:" + minute);
System.out.println("当前秒数:" + second);
// 添加或减去一定的天数、小时、分钟或秒数
LocalDateTime newDateTime = now.plusDays(1).plusHours(2).plusMinutes(30).minusSeconds(10);
System.out.println("修改后的日期和时间:" + newDateTime);
}
}
```
Java LocalDateTime
LocalDateTime是Java 8中引入的新的日期时间API,用于表示不带时区的日期和时间。它提供了更加直观和易用的日期时间操作方法,相比之前的Date和Calendar类,使用起来更加方便。
LocalDateTime的主要特点如下:
1. 不可变:LocalDateTime、LocalDate、LocalTime都是不可变对象,修改这些对象会返回一个新的副本,而不是在原对象上修改。
2. 带时区信息:LocalDateTime包含了年、月、日、时、分、秒、纳秒等信息,同时还带有时区信息,可以精确到毫秒级别。
3. 日期时间转换:LocalDateTime提供了丰富的方法来进行日期时间的转换,例如将LocalDateTime转换为Date、String等。
4. 日期时间操作:LocalDateTime提供了丰富的方法来进行日期时间的加减操作,例如加上或减去天数、小时数、分钟数等。
以下是一些常用的LocalDateTime操作示例:
```java
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class LocalDateTimeDemo {
public static void main(String[] args) {
// 获取当前时间
LocalDateTime now = LocalDateTime.now();
System.out.println("当前时间:" + now);
// 格式化时间
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"); String formattedNow = now.format(formatter);
System.out.println("格式化后的时间:" + formattedNow);
// 日期时间加减操作
LocalDateTime tomorrow = now.plusDays(1);
System.out.println("明天的时间:" + tomorrow);
LocalDateTime yesterday = now.minusDays(1);
System.out.println("昨天的时间:" + yesterday);
LocalDateTime sameDay = now.withHour(12).withMinute(0).withSecond(0);
System.out.println("中午12点整的时间:" + sameDay);
}
}
```<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
相关推荐
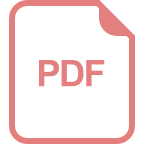
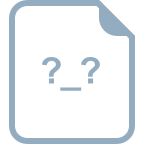












