ros中小海龟移动到指定位置后停留5秒继续下次运动
时间: 2024-10-07 16:03:09 浏览: 32

ros小海龟定点移动(程序)

在Robot Operating System (ROS) 中,小海龟(Turtlebot)通常使用`turtlebot_navigation`或`move_base`等包来进行路径规划和导航。要让小海龟移动到指定位置并暂停一段时间再执行下一项动作,你可以使用`move_base`节点中的`MoveBaseAction`和`actionlib`库。这里是一个简单的示例,说明如何实现这个功能:
```cpp
#include <ros/ros.h>
#include <move_base_msgs/MoveBaseAction.h>
#include <geometry_msgs/PoseStamped.h>
class TurtlebotController {
public:
explicit TurtlebotController(ros::NodeHandle &nh)
: actionClient_(nh, "move_base") {}
void moveToAndHold(const geometry_msgs::PoseStamped &goal, const int hold_time_seconds)
{
// 创建一个目标位置消息
move_base_msgs::MoveBaseGoal goal_msg;
goal_msg.target_pose = goal;
// 发送目标到行动服务器
actionClient_.send_goal(goal_msg);
// 等待到达目标点
if (!actionClient_.wait_for_result(std::chrono::seconds(hold_time_seconds))) {
ROS_ERROR("Timed out waiting for the move base action server.");
return;
}
// 检查结果
auto result = actionClient_.get_result();
if (!result->succeeded()) {
ROS_ERROR("Failed to reach target pose: %s", result->error_text.c_str());
return;
}
ROS_INFO("Successfully reached and held at the position for %d seconds.", hold_time_seconds);
}
private:
actionlib::SimpleActionClient<move_base_msgs::MoveBaseAction> actionClient_;
};
int main(int argc, char **argv)
{
ros::init(argc, argv, "turtlebot_controller");
ros::NodeHandle nh;
// 创建小海龟控制器实例
TurtlebotController turtlebot(nh);
// 定义目标位置和暂停时间(例如,(0, 0)坐标,暂停5秒)
geometry_msgs::PoseStamped goal;
goal.header.frame_id = "map"; // 使用适当的帧
goal.pose.position.x = 0.0;
goal.pose.position.y = 0.0;
goal.pose.orientation.w = 1.0; // 假设我们用四元数表示姿态
// 移动到并保持位置
turtlebot.moveToAndHold(goal, 5);
// ROS 主循环结束前关闭客户端
ros::spin();
return 0;
}
```
在这个例子中,你需要根据实际的机器人配置调整`header.frame_id`和坐标。`hold_time_seconds`参数设置为5,小海龟会在目标位置停留5秒。
阅读全文
相关推荐
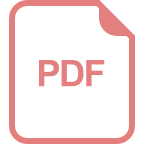
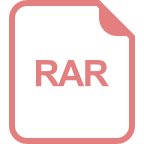


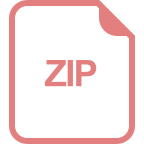
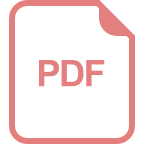
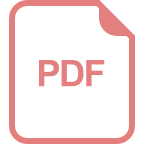
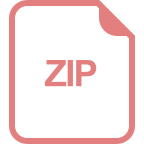
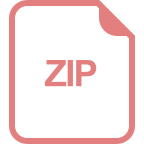
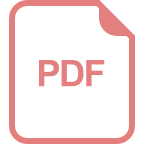
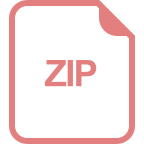
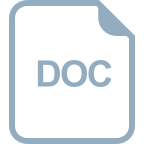
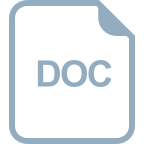

