多目标粒子群算法python代码
时间: 2024-01-01 20:23:17 浏览: 93
根据提供的引用内容,以下是一个使用Python实现的多目标粒子群算法的示例代码:
```python
import random
class Particle:
def __init__(self, num_objectives, num_dimensions):
self.position = [random.uniform(0, 1) for _ in range(num_dimensions)]
self.velocity = [random.uniform(0, 1) for _ in range(num_dimensions)]
self.best_position = self.position[:]
self.objectives = [0] * num_objectives
def update_velocity(self, global_best_position, inertia_weight, cognitive_weight, social_weight):
for i in range(len(self.velocity)):
cognitive_component = cognitive_weight * random.uniform(0, 1) * (self.best_position[i] - self.position[i])
social_component = social_weight * random.uniform(0, 1) * (global_best_position[i] - self.position[i])
self.velocity[i] = inertia_weight * self.velocity[i] + cognitive_component + social_component
def update_position(self):
for i in range(len(self.position)):
self.position[i] += self.velocity[i]
def evaluate_objectives(self, objective_functions):
self.objectives = [objective_function(self.position) for objective_function in objective_functions]
class MOPSO:
def __init__(self, num_particles, num_objectives, num_dimensions, num_iterations, inertia_weight, cognitive_weight, social_weight):
self.num_particles = num_particles
self.num_objectives = num_objectives
self.num_dimensions = num_dimensions
self.num_iterations = num_iterations
self.inertia_weight = inertia_weight
self.cognitive_weight = cognitive_weight
self.social_weight = social_weight
self.particles = []
self.global_best_position = [random.uniform(0, 1) for _ in range(num_dimensions)]
self.objective_functions = []
def add_objective_function(self, objective_function):
self.objective_functions.append(objective_function)
def initialize_particles(self):
for _ in range(self.num_particles):
particle = Particle(self.num_objectives, self.num_dimensions)
self.particles.append(particle)
def update_global_best_position(self):
for particle in self.particles:
if all(particle.objectives[i] < self.particles[0].objectives[i] for i in range(self.num_objectives)):
self.global_best_position = particle.position[:]
def run(self):
self.initialize_particles()
for _ in range(self.num_iterations):
for particle in self.particles:
particle.evaluate_objectives(self.objective_functions)
particle.update_velocity(self.global_best_position, self.inertia_weight, self.cognitive_weight, self.social_weight)
particle.update_position()
if all(particle.objectives[i] < particle.best_position[i] for i in range(self.num_objectives)):
particle.best_position = particle.position[:]
self.update_global_best_position()
# 示例使用的目标函数
def objective_function1(position):
return position[0] ** 2
def objective_function2(position):
return (position[0] - 2) ** 2
mopso = MOPSO(num_particles=30, num_objectives=2, num_dimensions=1, num_iterations=100, inertia_weight=0.5, cognitive_weight=1, social_weight=1)
mopso.add_objective_function(objective_function1)
mopso.add_objective_function(objective_function2)
mopso.run()
```
阅读全文
相关推荐

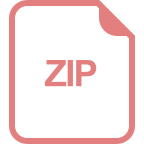
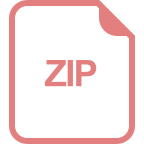











