java netty实现客户端和服务端长连接的完整代码
时间: 2024-12-14 20:23:57 浏览: 12
在Java中,Netty是一个高性能的网络应用框架,非常适合实现客户端和服务端的长连接。以下是一个简单的Netty示例,展示了如何创建一个TCP服务器和客户端进行全双工通信:
**服务端(Server.java):**
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class Server {
public static void main(String[] args) throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new LineBasedFrameDecoder(1024));
ch.pipeline().addLast(new StringDecoder());
ch.pipeline().addLast(new SimpleServerHandler());
}
});
ChannelFuture f = b.bind(8080).sync();
System.out.println("Server started and listening on port " + f.channel().localAddress());
// Keep the server running until interrupted.
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
// ... (定义SimpleServerHandler类处理客户端消息)
```
**客户端(Client.java):**
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
public class Client {
public static void main(String[] args) throws Exception {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new LineBasedFrameEncoder(1024));
ch.pipeline().addLast(new StringDecoder());
ch.pipeline().addLast(new SimpleClientHandler());
}
});
ChannelFuture f = b.connect("localhost", 8080).sync();
SocketChannel channel = (SocketChannel) f.channel();
channel.writeAndFlush("Hello Server!");
// Read and process messages from the server.
String response = "";
byte[] data = new byte[1024];
while ((response = channel.readBytes(data)) > 0) {
System.out.println("Received: " + new String(data, 0, response));
}
// Gracefully close the connection when done.
channel.close();
} finally {
group.shutdownGracefully();
}
}
}
// ... (定义SimpleClientHandler类发送请求并处理响应)
```
在这个例子中,`LineBasedFrameDecoder`用于拆分行,`StringDecoder`将字节流转换为字符串。`SimpleServerHandler`和`SimpleClientHandler`是自定义处理器,用于服务器和客户端的实际业务逻辑。
阅读全文
相关推荐
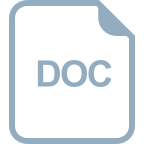
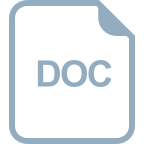
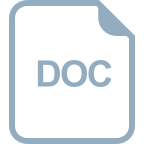


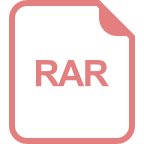
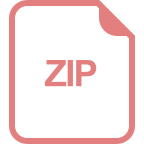
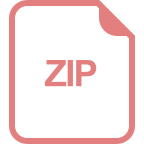
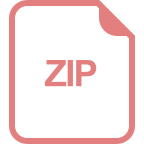
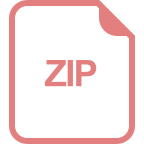







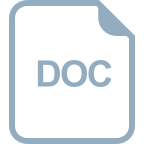