10个评委给选手打分,去掉一个最高分,去掉一个最低分,输出有效得分及计算选手的平均分用python写出代码
时间: 2024-11-22 15:40:30 浏览: 43
当然,我们可以使用Python编写一段简单的代码来实现这个需求。这里假设评分列表已经存储在一个名为`scores`的变量里,它是一个包含10个元素的列表,每个元素表示一个评委的分数。
```python
# 假设原始评分列表
scores = [85, 90, 92, 87, 95, 93, 88, 96, 89, 91]
# 首先,我们需要找到最高分和最低分
max_score = max(scores)
min_score = min(scores)
# 删除最高分和最低分
valid_scores = [score for score in scores if score != max_score and score != min_score]
# 计算有效得分的总和
total_valid_score = sum(valid_scores)
# 计算平均分
average_score = total_valid_score / len(valid_scores)
# 打印结果
print("有效得分:", total_valid_score)
print("选手的平均分:", average_score)
```
这段代码首先找出最高分和最低分,然后创建一个新的列表`valid_scores`,只包含介于剩余分数之间的值。接着,我们计算这些有效得分的总和和平均值并打印出来。
相关问题
输入评委打分10个评委给选手打分,去掉一个最高分,去掉一个最低分,输出有效得分及计算选手的平均分用python写出代
当然,我们可以使用Python来实现这个功能。首先,我们需要一个列表来存储所有评委的分数,然后按照指定的方式去除最高分和最低分,最后计算剩余有效得分的平均值。以下是一个简单的示例:
```python
def calculate_score(scores):
# 去除最高分和最低分
sorted_scores = sorted(scores)
highest_score = sorted_scores.pop() # 最高分
lowest_score = sorted_scores.pop(0) # 最低分
# 计算有效得分
effective_scores = [score for score in sorted_scores]
# 平均分计算
if len(effective_scores) > 0:
average_score = sum(effective_scores) / len(effective_scores)
else:
average_score = None # 如果没有有效得分,则返回None
return effective_scores, average_score
# 示例数据
scores = [85, 90, 78, 92, 88, 80, 95, 86, 83, 89]
effective_scores, average_score = calculate_score(scores)
print(f"有效得分: {effective_scores}")
print(f"选手平均分: {average_score}")
#
python十名评委给选手打分去掉最高分和最低分计算平均分
好的,可以使用Python编写一个函数实现这个功能,代码如下:
```python
def calculate_score(scores):
# 对分数进行排序
sorted_scores = sorted(scores)
# 去掉最高分和最低分
trimmed_scores = sorted_scores[1:-1]
# 计算平均分
avg_score = sum(trimmed_scores) / len(trimmed_scores)
return avg_score
```
这个函数接受一个评委打分的列表作为参数,返回去掉最高分和最低分后的平均分数。使用时,可以将十个评委的分数放到一个列表中,然后调用这个函数即可,例如:
```python
scores = [90, 85, 92, 88, 89, 91, 87, 86, 93, 94]
avg_score = calculate_score(scores)
print("去掉最高分和最低分后的平均分数为:", avg_score)
```
输出结果为:
```
去掉最高分和最低分后的平均分数为: 89.0
```
这表示这位选手的最终得分为89分。
阅读全文
相关推荐
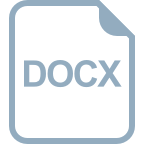
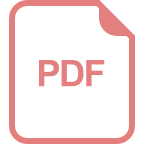













