vuex在vue2上控制一个面板上不同组件的现实,一次只能显示一个
时间: 2024-09-11 16:12:35 浏览: 72
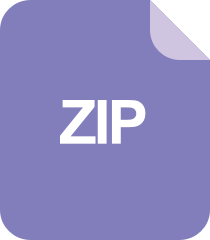
一个基于 Vue3.2 +ElementPlus的思维导图.zip
Vuex 是一个专为 Vue.js 应用程序开发的状态管理模式和库。它采用集中式存储管理应用的所有组件的状态,并以相应的规则保证状态以一种可预测的方式发生变化。在 Vue2 中使用 Vuex 来控制一个面板上不同组件的显示,可以通过以下步骤实现:
1. 安装并引入 Vuex:首先需要在项目中安装 Vuex,并在应用的入口文件中引入。
2. 创建 Vuex store:创建一个 store 文件来集中管理应用的状态。在这个例子中,状态可以包括当前显示的组件的标识。
3. 定义状态(state):在 store 中定义一个状态,比如 `activePanel`,用于记录当前激活的面板组件标识。
4. 定义 mutations:创建 mutations 来更新 `activePanel` 的值。这是改变状态的唯一途径,确保状态的改变是可追踪的。
5. 创建组件:为面板上的每个组件创建对应的 Vue 组件。
6. 绑定组件与 store:在每个组件的 `mounted` 钩子或者通过事件触发的方式,调用 commit 提交 mutation 来更新 `activePanel` 的值。
7. 使用计算属性:在面板的显示区域,使用计算属性来根据 `activePanel` 的值决定显示哪个组件。
以下是一个简单的代码示例:
```javascript
// store.js
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export default new Vuex.Store({
state: {
activePanel: 'componentA' // 默认显示第一个组件
},
mutations: {
SET_ACTIVE_PANEL(state, panel) {
state.activePanel = panel;
}
},
actions: {
setActivePanel({ commit }, panel) {
commit('SET_ACTIVE_PANEL', panel);
}
}
});
// componentA.vue
<template>
<div>Component A</div>
</template>
<script>
export default {
mounted() {
// 触发store中的mutation来激活此组件
this.$store.dispatch('setActivePanel', 'componentA');
}
};
</script>
// componentB.vue
<template>
<div>Component B</div>
</template>
<script>
export default {
mounted() {
// 触发store中的mutation来激活此组件
this.$store.dispatch('setActivePanel', 'componentB');
}
};
</script>
// panel.vue (面板显示区域)
<template>
<div>
<component-a v-if="activePanel === 'componentA'"></component-a>
<component-b v-else-if="activePanel === 'componentB'"></component-b>
<!-- 更多组件... -->
</div>
</template>
<script>
export default {
computed: {
activePanel() {
return this.$store.state.activePanel;
}
}
};
</script>
```
通过上述方式,你可以控制不同组件在面板上的显示,一次只显示一个组件。
阅读全文
相关推荐
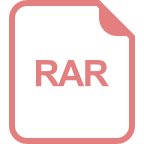
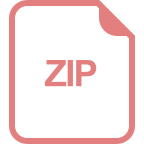
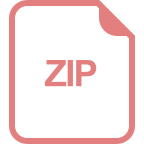
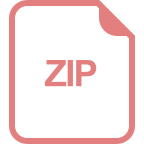
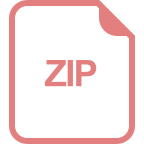
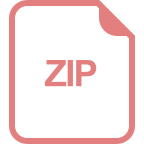
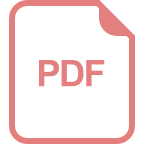
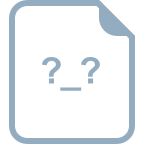
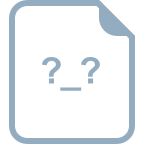
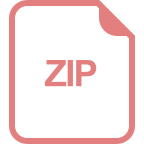
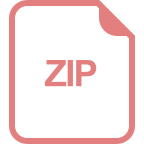
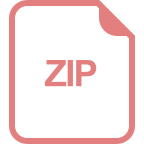
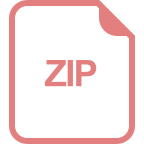
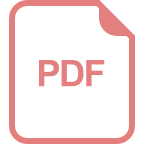
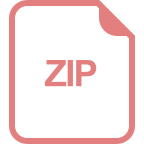
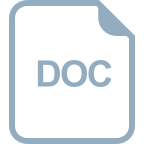
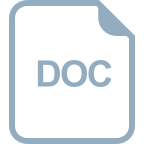
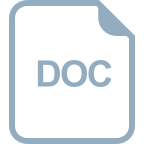