输入时分秒的一个具体时间,要求打印输出它的下一秒,如何用Java编写程序
时间: 2024-09-12 22:07:44 浏览: 19
要用Java编写程序来处理输入的时间,并打印出下一秒的时间,可以按照以下步骤进行:
1. 首先,需要获取用户输入的时、分、秒。
2. 然后,根据输入创建一个`LocalTime`对象。
3. 接着,给这个对象加一秒。
4. 最后,格式化输出这个时间对象。
下面是一个简单的Java程序示例,演示了如何实现这个功能:
```java
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
import java.util.Scanner;
public class NextSecond {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入时间(格式如HH:mm:ss):");
String input = scanner.nextLine();
// 解析输入的时间字符串
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("HH:mm:ss");
LocalTime localTime = LocalTime.parse(input, formatter);
// 增加一秒
LocalTime nextSecond = localTime.plusSeconds(1);
// 输出下一秒的时间
System.out.println("下一秒的时间是:" + nextSecond.format(formatter));
}
}
```
这段代码首先通过`Scanner`类获取用户输入的时间字符串,然后使用`DateTimeFormatter`来解析这个字符串。之后,创建一个`LocalTime`对象,并使用`plusSeconds`方法来获取下一秒的时间。最后,再次使用`DateTimeFormatter`将`LocalTime`对象格式化输出。
相关推荐
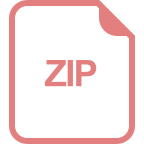
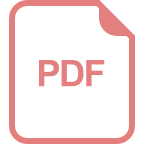
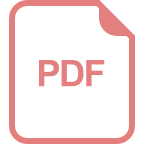














