设计一个时间类,包含时分秒数据的初始化,并编写友元函数输出一个时间对象的值。 输入 输入一个时间的时分秒 输出 输出时间的时分秒
时间: 2024-05-04 21:16:48 浏览: 75
以下是一个简单的时间类的设计,包含时分秒数据的初始化,并编写友元函数输出一个时间对象的值:
```c++
#include <iostream>
using namespace std;
class Time {
private:
int hour, minute, second;
public:
Time(int h = 0, int m = 0, int s = 0) : hour(h), minute(m), second(s) {}
friend void printTime(Time t);
};
void printTime(Time t) {
cout << "Time is: " << t.hour << ":" << t.minute << ":" << t.second << endl;
}
int main() {
Time t(12, 34, 56);
printTime(t);
return 0;
}
```
可以看到,我们定义了一个叫做 Time 的类,其中包含了 hour、minute 和 second 三个私有成员变量,分别表示时间的时、分、秒。构造函数支持传入时、分、秒的参数进行初始化。我们还定义了一个友元函数 printTime,用于输出一个时间对象的值。在 main 函数中,我们创建了一个时间对象 t,并将其作为参数传入 printTime 函数中进行输出。
相关问题
用一个友元函数实现以12小时的方式输出时间
在C++中,友元(Friend Function)是一种特殊的关系,允许非成员函数直接访问类的私有和保护成员,而无需创建对象实例。如果你想用一个友元函数以12小时制的方式输出时间,你可以这样做:
首先,定义一个包含时间数据的类,如`Time`:
```cpp
class Time {
private:
int hours;
int minutes;
public:
// 构造函数、getters等...
};
// 定义友元函数,注意友元函数前加上friend关键字
friend std::string format12Hour(const Time& time);
```
然后,在`format12Hour`友元函数中实现12小时格式的转换:
```cpp
std::string format12Hour(const Time& time) {
if (time.hours > 12) {
return std::to_string(time.hours - 12) + " PM";
} else if (time.hours == 0) { // 处理 AM 和 PM 的边界情况
return "12 AM";
} else {
return std::to_string(time.hours) + " AM";
}
// 可能需要处理分钟的情况,比如格式化为"2:30 PM"
}
```
当你有一个`Time`对象的时候,可以直接通过类名调用这个友元函数来获取12小时格式的时间字符串。
用一个友元函数实现以24小时的方式输出时间
在C++中,你可以通过将一个成员函数声明为另一个类的友元函数来实现这个功能。友元函数可以访问类的私有和保护成员,这对于某些需要直接操作类内部状态的情况非常有用。以下是一个简单的例子,假设有一个`TimeOfDay`类,它有私有的`hour`, `minute`, 和 `second` 变量表示一天中的时刻,我们可以创建一个友元函数`formatTime()`来以24小时制格式输出时间:
```cpp
class TimeOfDay {
private:
int hour;
int minute;
int second;
public:
// 构造函数和其他成员函数...
// 定义友元函数
friend std::string formatTime(const TimeOfDay& time);
};
// 友元函数实现
std::string formatTime(const TimeOfDay& time) {
return std::to_string(time.hour < 10 ? "0" : "") + std::to_string(time.hour) +
":";
// 类似的处理分钟和秒,这里只展示了小时部分
}
// 使用示例
int main() {
TimeOfDay t(15, 30, 0); // 创建一个下午3点30分的时间
std::string timeStr = formatTime(t);
std::cout << "The time is: " << timeStr << std::endl; // 输出: The time is: 15:30
return 0;
}
```
阅读全文
相关推荐
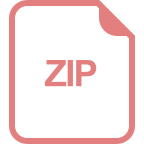
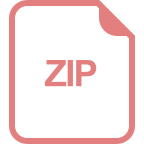
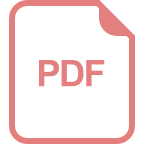













