用C++设计一个complex类并用友元函数重载乘法运算符
时间: 2024-02-11 18:06:03 浏览: 90
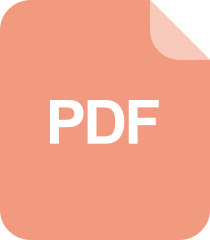
C++运算符重载函数作为类成员函数和友元函数.pdf
好的,下面是实现代码:
```c++
#include <iostream>
using namespace std;
class complex {
private:
double real;
double imag;
public:
complex(double r = 0, double i = 0) : real(r), imag(i) {}
friend complex operator*(const complex &c1, const complex &c2);
void print() {
cout << "(" << real << "," << imag << ")" << endl;
}
};
complex operator*(const complex &c1, const complex &c2) {
double r = c1.real * c2.real - c1.imag * c2.imag;
double i = c1.real * c2.imag + c1.imag * c2.real;
return complex(r, i);
}
int main() {
complex c1(1, 2), c2(3, 4);
complex c3 = c1 * c2;
c3.print();
return 0;
}
```
解释一下:
- `complex` 类表示复数,有实部和虚部两个数据成员;
- 构造函数用于初始化实部和虚部;
- `operator*` 是友元函数,用来重载乘法运算符;
- `print` 函数用于输出复数。
在 `main` 函数中,创建两个复数 `c1` 和 `c2`,并计算它们的积 `c3`,最后输出 `c3`。
阅读全文
相关推荐
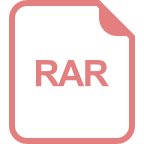
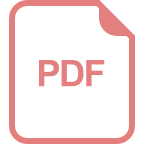






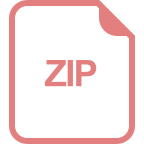
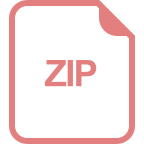
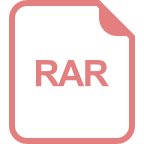
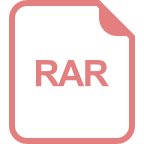
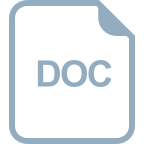
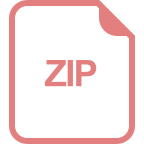
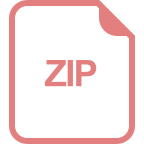